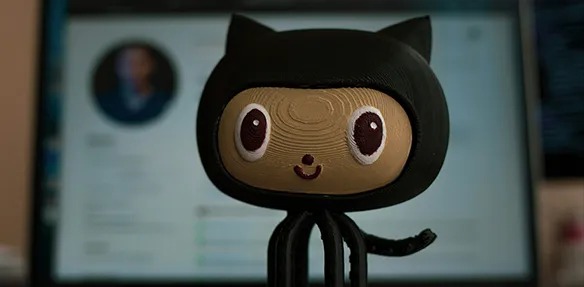
Git rebase and merge are two ways to combine branch changes. Here’s what you need to know:
- Git merge: Keeps all commit history, creates non-linear timeline
- Git rebase: Rewrites commit history for a linear timeline
Quick comparison:
Feature | Git Merge | Git Rebase |
---|---|---|
History | Preserves | Rewrites |
Timeline | Non-linear | Linear |
Ease of use | Simpler | More complex |
Best for | Public branches, big teams | Local branches, small teams |
When to use each:
-
Use merge for:
- Public branches
- Large team collaboration
- Preserving full change context
-
Use rebase for:
- Local feature branches
- Clean, linear history
- When you’re OK rewriting history
Remember: Never rebase public branches. It’s a recipe for team headaches.
Stuck? Go with merge. It’s safer and easier to undo.
This article covers how rebase and merge work, when to use each, their pros and cons, and tips for using them effectively. We’ll also look at team strategies and common Git mistakes to avoid.
What is Git Rebase?
Git rebase is a tool that keeps your project history clean. It’s one of two main ways to combine changes from one branch to another in Git.
Definition of Git Rebase
Git rebase moves a series of commits to a new base commit. It’s like saying, “Let’s put my changes on top of what everyone else has done.”
Here’s what happens:
- Git removes your current branch’s commits
- It applies the new base commit
- It replays your commits on top
This creates new commits for each original commit, rewriting your project history.
How Rebase Works
Let’s break it down:
- You’re on your feature branch and run
git rebase master
- Git finds where your branch and master split
- It saves your branch’s changes as temporary patches
- Your branch is reset to match master
- Git applies each patch one by one
If there are conflicts, Git pauses for you to fix them. After that, use git rebase --continue
to keep going.
When to Use Rebase
Rebase is great for:
- Updating feature branches
- Cleaning up local commits
- Creating a linear project history
But remember: NEVER rebase commits you’ve pushed to a public repo. It’ll cause problems for your team.
“Don’t rebase branches you’ve shared with others. Rebase is for cleaning up local commits. Once commits are public, treat them as set in stone.” — Atlassian Git Tutorial
What is Git Merge?
Git merge combines changes from one branch into another. It’s how developers integrate work from different branches into the main codebase.
Definition of Git Merge
Git merge takes content from a source branch and integrates it with a target branch. It creates a new commit on the target branch that links the histories of both branches.
Unlike rebase, merging keeps the entire history of both branches. This makes tracking changes easier.
How Merge Works
Here’s how merge works:
- Git finds the common ancestor commit of the two branches.
- It compares changes between this point and the tips of both branches.
- Git automatically combines non-conflicting changes.
- A new commit is created with all the changes.
If there are conflicts, Git pauses and asks you to fix them manually.
When to Use Merge
Merge is great for:
- Adding completed features to the main branch
- Team collaboration
- Keeping a clear record of changes
“Merging is better for handling conflicts, as it presents them all at once, making it easier to manage.” - Git Documentation
Merge vs. Rebase:
Scenario | Merge | Rebase |
---|---|---|
Public branches | ✓ | ✗ |
Preserving history | ✓ | ✗ |
Linear history | ✗ | ✓ |
Frequent integration | ✓ | ✗ |
Rebase vs. Merge: Key Differences
Git rebase and merge both integrate changes from one branch to another. But they work differently.
Main Differences
-
History: Merge keeps original history. Rebase rewrites it.
-
Commit Structure: Merge adds a new commit. Rebase moves existing commits.
-
Workflow: Merge is safer for shared work. Rebase is better for personal branches.
Pros and Cons
Aspect | Git Merge | Git Rebase |
---|---|---|
History | Full | Linear |
Traceability | Easy | Can be tricky |
Collaboration | Good for teams | Good for solo work |
Conflicts | All at once | One by one |
Risk | Lower | Higher |
Team Impact
Merge shines in team settings:
- Safe for public branches
- Great for group work
- Keeps accurate history
Rebase can clean up history but might complicate teamwork. Use it:
- On your own branches
- Before sharing your work
- To tidy up commits
“Rebase solo branches. Merge team branches.” - Git Best Practices Guide
Choose wisely based on your project needs and team setup.
5 Times to Use Git Rebase
Git rebase can clean up your commit history. Here’s when to use it:
When to Rebase
- Updating feature branches
Your feature branch is behind main? Catch up with:
git checkout feature-branch
git rebase main
- Cleaning local commits
Tidy up before pushing:
git rebase -i HEAD~5
- Integrating upstream changes
Working on a fork? Get updates from the original:
git pull --rebase upstream main
- Squashing commits
Turn small commits into one big one:
git rebase -i HEAD~3
- Reordering commits
Make your commits logical before merging:
git rebase -i HEAD~5
Rebase Safely
- Rebase BEFORE pushing to shared repos
- Use
git push --force-with-lease
instead ofgit push -f
- Talk to your team when rebasing shared branches
- Keep rebases small and frequent
Rebase Risks and Fixes
Risk | Fix |
---|---|
Lost commits | Use git reflog to get them back |
Conflicts | Solve one by one with git rebase --continue |
Duplicate commits | Check git log before and after rebasing |
Broken builds | Run tests after rebasing |
5 Times to Use Git Merge
Git merge is your go-to for combining work from different branches. Here’s when to use it:
- Finishing a feature
Your feature’s ready? Bring it home:
git checkout main
git merge feature-branch
This pulls your work into main, keeping the full history.
- Getting team updates
Sync with your colleagues:
git checkout your-branch
git merge origin/main
- Prepping for release
Combine features for release:
git checkout release-1.0
git merge feature-a feature-b feature-c
- Fixing urgent issues
Quick fixes for production:
git checkout main
git merge hotfix-branch
- Keeping long-term branches in sync
Align parallel development:
git checkout experimental
git merge main
Merge Like a Pro
- Pull latest changes first
- Use
--no-ff
for a merge commit - Write clear merge messages
- Review before finalizing
Dealing with Merge Conflicts
When Git can’t auto-merge:
- Open conflicting files
- Find conflict markers
- Fix conflicts
- Stage fixed files
- Commit the merge
For tricky conflicts, try:
git mergetool
This visual tool can make your life easier.
Team Size and Git Strategy
Your team size affects your Git strategy. Let’s look at how.
Small vs. Large Teams
Small teams (2-6 devs) often do this:
- New branch for each task
- Rebase to stay current with main
- Merge only into main via pull requests
This keeps history clean and easy to follow.
Larger teams usually prefer merging:
- Multiple devs on parallel features
- Less risky than rebasing
- Easier to track feature progress
Atlassian’s Stash team, for example, only merges. They use pull requests for all features, ensuring quality and review.
Team Git Rules
Clear Git rules help everyone stay on the same page. Consider these:
- Branch names
Use ticket numbers and brief descriptions:
feature/TICKET-123-add-login-page
bugfix/TICKET-456-fix-memory-leak
- Commit messages
Stick to a format:
[TICKET-123] Add login page
- Implement user auth
- Create login form UI
- Add error handling
- Code reviews
Set clear review rules:
- At least one approval to merge
- Max review time (e.g., 24 hours)
- Auto-checks for style and tests
- Merge vs. Rebase
Choose a team policy:
Policy | Best For | Notes |
---|---|---|
Merge-only | Big teams, complex projects | Keeps full history, easier conflicts |
Rebase-then-merge | Small teams, clean history | Needs Git expertise |
Hybrid | Mid-size teams | Rebase small changes, merge big features |
- Branch lifecycle
Set branch rules:
- Delete feature branches after merging
- Clean up old branches (e.g., 30+ days)
Advanced Git Techniques
Git’s got some cool tricks up its sleeve. Let’s dive into a few that’ll make you a Git wizard.
Interactive Rebase: Your Commit Time Machine
Ever wish you could rewrite history? With interactive rebase, you can:
git rebase -i HEAD~3
This opens a playground where you can shuffle commits, fix typos, or even make commits disappear. It’s like editing a movie before it hits theaters.
“Interactive rebase is like having a time machine for your commits.” - Git Guru
Cherry-Pick: Plucking the Best Commits
Cherry-picking is like being a code DJ. You can grab the best bits from one branch and drop them into another:
git cherry-pick <commit-hash>
It’s perfect for when you need that one bug fix, but don’t want the whole branch.
The Rebase-Merge Tango
Want a clean, straight line in your Git history? Try this dance:
- Rebase your feature branch onto main
- Merge it back
It’s like ironing your clothes before putting them on. Everything looks neat and tidy.
Technique | Use Case | Why It’s Awesome |
---|---|---|
Interactive Rebase | Pre-sharing cleanup | Makes your history look pro |
Cherry-Pick | Selective changes | Grabs just what you need |
Rebase + Merge | Feature integration | Keeps things linear and clean |
These techniques aren’t just fancy tricks. They’re power tools for keeping your codebase manageable. Use them wisely, and your future self will thank you.
Common Git Mistakes to Avoid
Git’s powerful, but it’s easy to mess up. Here are some pitfalls and how to dodge them:
Don’t Rebase Public Branches
Rebasing shared branches? Bad idea. Here’s why:
- Rewrites history, confusing your team
- Can create duplicate commits
- Breaks the “don’t change shared history” rule
Stick to merging for public branches. Save rebases for your local work.
Handling Tough Merge Conflicts
Merge conflicts happen. Deal with them like this:
- Break them into smaller parts
- Use visual diff tools
- Talk to your team about complex merges
“For tough merges, step back and plan. Smaller chunks are easier to handle”, says Git creator Linus Torvalds.
Keeping Long Branches Updated
Long feature branches can drift from main. To prevent this:
- Pull from main often
- Use feature flags for long-term work
- Split big features into smaller, mergeable pieces
Mistake | Result | Fix |
---|---|---|
Rebasing public branches | Confusion, conflicts | Merge instead |
Ignoring conflicts | Broken code, lost work | Address promptly |
Stale branches | Hard merges later | Update from main regularly |
Conclusion
Git rebase and merge are two ways to combine branch changes. Here’s what you need to know:
Git merge keeps all commit history, creating a non-linear timeline. It’s easier but can clutter history.
Git rebase rewrites commit history for a linear timeline. It’s cleaner but needs careful use.
Feature | Git Merge | Git Rebase |
---|---|---|
History | Keeps all commits | Rewrites history |
Timeline | Non-linear | Linear |
Ease of use | Simpler | More complex |
Best for | Public branches, big teams | Local branches, small teams |
Picking Your Git Strategy
Choose based on your project:
Use merge for:
- Public branches
- Large team collaboration
- Preserving full change context
Use rebase for:
- Local feature branches
- Clean, linear history
- When you’re OK rewriting history
You can mix both. Try rebase for local work and merge for main branch integration.
“Commit to the main branch often to avoid long-lived branch headaches.”
This DevOps advice shows a shift: moving from complex workflows like Gitflow to simpler, trunk-based development.
Learn More About Git
Want to master Git, especially rebasing and merging? Here’s where to start:
Official Git Resources
Git’s got your back with free, in-depth learning materials:
- Git Docs: Detailed command explanations
- Pro Git Book: Free online book covering everything Git
See Git in Action
These tools make Git concepts crystal clear:
Tool | What It Does | Perfect For |
---|---|---|
Oh My Git | Git as a game | Newbies |
Learn Git Branching | Interactive commit tree | Branching pros |
Explain Git with D3 | CLI with instant graphs | Command explorers |
GitKraken | GUI with clear history | Students (free plan) |
GitUp | Free, open-source GUI | Mac fans |
Want hands-on practice? Try Git Gud or Git School. Run commands, see results.
Level Up Your Skills
Check out these courses:
- Google’s “Intro to Git and GitHub” (16 hours, free audit)
- Udacity’s “Version Control with Git” (9 hours)
- University of Manchester’s “Collaborative Coding with Git” (12 hours)
FAQs
When to use git rebase instead of merge?
Git rebase and merge serve different purposes. Here’s a quick guide:
Scenario | Use | Why |
---|---|---|
Private branch | Rebase | Cleaner history |
Shared branch | Merge | Keeps original commits |
Full history needed | Merge | All commits intact |
Simplify graph | Rebase | Less complex |
Don’t rebase public branches. It’s a recipe for team headaches.
Stuck? Go with merge. It’s safer and easier to undo.
For trickier situations:
- Updating feature branches
Rebase to sync with main. Fewer conflicts later.
- Pre-merge cleanup
Rebase to tidy up commits before merging to main.
- Handling conflicts
Merge: One-time conflict resolution. Rebase: Might need fixes for each commit.