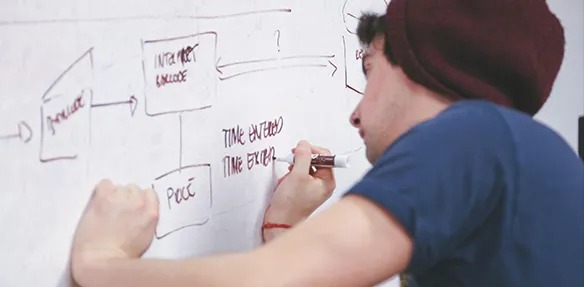
API versioning is crucial for maintaining stability while innovating. Here’s what you need to know:
- Use semantic versioning (major.minor.patch)
- Keep backward compatibility when possible
- Communicate changes clearly to users
- Support multiple versions simultaneously
- Test thoroughly across all versions
- Use tools like Git, API gateways, and testing suites
Key practices:
- Plan versioning from the start
- Use clear version numbering
- Maintain old versions
- Provide detailed changelogs
- Give ample notice before retiring versions
- Prioritize security for all versions
- Conduct extensive testing
- Leverage appropriate tools
Remember: Good API versioning builds trust, enables innovation, and keeps your service stable.
Quick Comparison:
Method | Pros | Cons | Used By |
---|---|---|---|
URL Path | Easy to use, simple testing | URL clutter | Facebook, Twitter |
Header | Clean URLs, precise control | Extra header work | Microsoft |
Query Parameter | Simple setup, optional | Can clutter URLs | Various APIs |
Content Type | Resource-level control | Complex, harder to test | GitHub |
Choose a method that fits your needs and stick with it for consistency.
API Versioning Basics
API versioning lets you update your API without breaking existing apps. It’s like giving your API a series of snapshots over time.
What is API Versioning
API versioning assigns different versions to an API as it changes. Each version is a snapshot of your API’s functionality at a specific point.
Think of it as a time machine for your API. Developers can choose which “moment” they want to use.
Here’s a simple example with a weather API:
- V1: Basic temperature data
- V2: Adds humidity info
- V3: Introduces wind speed
Versioning ensures apps using V1 don’t break when you add new features in V2 or V3.
Main Version Types
The industry standard is Semantic Versioning. It uses a three-part number: Major.Minor.Patch.
-
Major Version: Big changes that aren’t backward-compatible. V1.0.0 to V2.0.0? Expect some breakage.
-
Minor Version: New features that don’t break existing stuff. V1.1.0 to V1.1.2? Should be smooth sailing.
-
Patch Version: Bug fixes and small tweaks. V1.0.1 to V1.0.2? Totally safe.
Stripe, a payment processing API, uses this system. They’re on version 2022-11-15, showing the date of the last major update.
When to Add Versions
Not sure when to create a new version? Here are some clear signs:
-
Breaking Changes: Changing response structure or altering existing endpoints? Time for a new major version.
-
Big New Features: Adding major functionality that doesn’t fit the current version? Consider a new minor version.
-
Removing Features: Planning to axe existing features? A new version gives users time to adapt.
-
Security Overhauls: Major security changes often need a new version to protect all users.
Remember, versioning isn’t just tech stuff - it’s communication. Each new version tells users about your API’s state and direction.
“APIs are forever.” - Werner Vogels, CTO of Amazon
This quote highlights why versioning matters. Your API is a long-term deal, and versioning helps you keep that promise while still moving forward.
Main Versioning Methods
API versioning isn’t a one-size-fits-all deal. Let’s look at the main approaches and see how they stack up.
URL Path Versions
This is the go-to method for big players like Facebook, Twitter, and Airbnb. Why? It’s simple: you slap the version number right in the URL.
https://api.example.com/v1/products
It’s easy to spot and test. But here’s the catch: new versions mean new URLs. Over time, your codebase might get a bit bloated.
Header Versions
Microsoft likes this one. Instead of messing with the URL, you tuck the version into a custom header.
GET /products HTTP/1.1
X-API-Version: 1.0
Your URLs stay clean, but developers need to remember to include that custom header.
Query Parameter Versions
Another straightforward approach: add the version as a URL parameter.
https://api.example.com/products?version=1.0
It’s easy to implement and makes versioning optional. But if you’re using lots of query parameters, things can get messy.
Content Type Versions
GitHub’s choice. It’s all about fine-tuning resource representations.
GET /products HTTP/1.1
Accept: application/vnd.myapi.v2+json
You can version individual resources, but it’s more complex and trickier to test.
Version Method Comparison
Here’s a quick rundown:
Method | Pros | Cons | Used By |
---|---|---|---|
URL Path | Easy to get, simple to test | URL overload | Facebook, Twitter, Airbnb |
Header | Clean URLs, precise control | Extra header work | Microsoft |
Query Parameter | Simple setup, optional | Can clutter URLs | Various APIs |
Content Type | Resource-level versioning | More complex, harder to test | GitHub |
Your choice depends on your needs. Think about ease of use, client compatibility, and your API’s complexity.
“Pick the versioning method that fits your API’s needs and stick with it. Consistency is key.” - API Design Best Practices
Using Semantic Versioning
Semantic Versioning (SemVer) is the go-to system for managing API updates. It’s a straightforward way to communicate changes and keep things running smoothly.
Major Changes
Major changes are the big ones. They break existing code and force users to update their stuff. In SemVer, we bump up the first number (1.0.0 to 2.0.0).
Take Stripe API, for example. In March 2023, they jumped from version 2022-11-15 to 2023-03-15. This update was a doozy - they axed old API versions and tweaked how some endpoints worked.
“We try to keep things backward compatible, but sometimes we gotta make big changes to improve the API”, said Michael Glukhovsky, Stripe’s lead developer.
Minor Updates
Minor updates add new goodies without breaking what’s already there. We increase the second number for these (1.1.0 to 1.2.0).
GitHub’s REST API is a pro at this. In their v3 API, they often toss in new endpoints or parameters without messing with the major version. For instance, in October 2022, they added some new fields to the “List repositories for a user” endpoint. No sweat for existing users.
Bug Fixes
Bug fixes and small performance boosts? Those are patch updates. They’re the safest changes, and we bump up the third number (1.0.1 to 1.0.2).
Twilio, the cloud communications folks, drops these regularly. In a recent update (2023-02-01), they squashed a bug in their SMS API that was causing some message delays. Users didn’t need to do a thing, but the service got more reliable.
Version Numbers 101
Here’s a quick cheat sheet for version numbers:
What Changed? | Which Number? | Example |
---|---|---|
Breaks Stuff | First (X.y.z) | 1.0.0 → 2.0.0 |
New Feature | Second (x.Y.z) | 1.1.0 → 1.2.0 |
Bug Fix | Third (x.y.Z) | 1.1.1 → 1.1.2 |
When you bump up a higher number, the lower ones reset to zero. So 1.9.3 becomes 2.0.0, not 2.9.3.
Breaking vs Non-Breaking Changes
Knowing the difference between breaking and non-breaking changes is key. Breaking changes force users to update their code. Non-breaking changes? They play nice with existing setups.
Breaking changes are things like:
- Killing or renaming endpoints
- Changing data types in existing fields
- Adding must-have parameters
Non-breaking changes include:
- Adding new optional parameters
- Introducing new endpoints
- Expanding what values a field can take
“The goal isn’t to make everything compatible. It’s about figuring out what matters most to your users and being clear about it”, says Ashwin Raghav Mohan Ganesh, who leads engineering for Project IDX at Google.
When in doubt, treat a change as breaking. It’s better to over-communicate than to surprise your users with a broken system.
Keeping Versions Compatible
Juggling multiple API versions is tricky. It’s about moving forward without breaking what works. Let’s look at how to keep your API versions playing nice together.
Backward Compatibility Rules
The big rule for API versioning? Don’t break existing stuff. Backward compatibility should guide every change you make.
Stripe does this well. In their March 2023 update, they added new features without messing up existing ones. How? They added optional parameters and new endpoints instead of changing old ones.
To keep things backward compatible:
- Make new fields optional
- Create new endpoints for big changes
- Don’t remove or rename existing parameters
- Keep old response structures the same
It’s always easier to add than to take away.
Removing Old Features
Sometimes you need to get rid of old features. But how do you do it without leaving users hanging?
Twitter’s approach is worth copying. When they retired their v1 API:
- They announced it way ahead of time (over a year)
They gave developers plenty of warning so they could prepare.
- They provided detailed migration guides
These guides helped developers understand what was changing and how to adapt.
- They offered support during the transition
Developers could get help if they ran into issues while updating their integrations.
The key? Clear communication and lots of time to adapt.
Update Paths
Getting users to newer versions isn’t easy. GitHub does this well with their REST API. They make updating smooth by:
- Offering detailed migration guides
- Providing code samples for new implementations
- Running old and new versions side by side during transitions
This lets developers upgrade when they’re ready, without feeling rushed.
End-of-Life Rules
You need clear rules for retiring old API versions. Twilio has a good approach:
- They announce end-of-life dates at least 12 months ahead
- They keep both old and new versions running for a while
- They offer extra support for big clients who need more time
This gives developers plenty of time to plan and make changes.
Managing Version Changes
Balancing new features with old version support is tough. Akshay Kothari from Notion says:
“We’re not trying to make everything compatible forever. We focus on what matters most to our users and communicate that clearly.”
This idea guides how they handle versioning, letting them innovate while keeping things stable for current users.
To manage version changes well:
- Use feature flags to roll out new stuff slowly
- Try A/B testing to see how users react to changes
- Keep detailed docs for each version
- Use API gateways to handle requests across different versions
Writing and Sharing Updates
Good API versioning hinges on solid communication. Here’s how to keep your users in the loop about API changes:
Change Logs
Change logs are your API’s history book. They list all tweaks and updates in order. Stripe nails this. Their logs are clear and packed with details.
Check out Stripe’s March 2023 update:
- New: “Added Apple Pay for web”
- Fixed: “Issue with some refunds not processing”
- Heads up: “We’re retiring the
create_customer
endpoint in the next big update”
Quick tip: Use semantic versioning. Big changes? Bump the first number (2.0.0). New features? Second number (2.1.0). Small fixes? Last number (2.0.1).
API Docs Updates
Fresh docs are as crucial as the changes themselves. GitHub’s a pro at this. They update their REST API docs with each release, flagging new stuff, changed bits, and soon-to-be-gone features.
When GitHub added new fields to their “List user repos” endpoint in October 2022, they:
- Highlighted the new fields
- Showed how to use them
- Updated related endpoints
Your docs are often a dev’s first stop. Keep them current and easy to use.
User Alerts
Heads-up communication saves devs headaches. Twilio’s got this down pat. They use multiple channels:
- Emails for big changes
- In-app alerts for urgent stuff
- Blog posts for deep dives
- Social media for wide reach
When Twilio planned to retire an old SMS API version in January 2023, they gave a six-month warning. Plenty of time for devs to adapt.
End-of-Life Notices
When it’s time to sunset an API version, be crystal clear. Salesforce does this well. Their notices usually include:
- The exact retirement date
- Other options for affected users
- Who to contact for help
Here’s how Salesforce laid out their SOAP API retirement in 2022:
When | What |
---|---|
Spring ‘22 | We’re retiring these versions |
Winter ‘23 | No more support |
Spring ‘24 | They’re gone for good |
This timeline gave devs two years to plan their move.
Version Support Schedule
A support schedule tells users how long they can count on each API version. Google’s Cloud APIs set a good example:
- They support each version for at least a year from release
- And for 3 months after a new version drops
Google puts this schedule right in their docs. It helps devs plan their work with confidence.
Testing Each Version
Testing is key when you’re juggling multiple API versions. It’s how you make sure everything works smoothly, stays compatible, and doesn’t break. Let’s break down the main parts of testing each API version.
Version Tests
Version tests make sure your API updates work right across different versions. You need to create solid test suites for each version and run them regularly.
Stripe, the payment processing company, nails this. In their March 2023 update, they:
- Ran tons of automated tests
They put over 10,000 automated tests through their paces across all API versions. This helped them catch any hiccups in existing features.
- Tested real-world scenarios
Stripe simulated how their API would work with popular e-commerce platforms and payment gateways.
- Looked for weird edge cases
Their team hunted down unusual scenarios that might break the API.
“We caught 37 potential issues before they hit production. That saved our developers a lot of headaches”, said David Singleton, Stripe’s Head of Engineering.
Checking Compatibility
You need to make sure new versions play nice with old ones. Developers hate it when things break. GitHub does this really well:
- They use automated tools to catch compatibility issues early.
- GitHub does “time travel” testing. They pretend to be old client libraries talking to newer API versions.
- They have a big set of tests that cover all supported versions.
In 2022, this approach helped GitHub catch and fix 89% of potential breaking changes before users even noticed.
Speed Tests
Nobody likes a slow API. Speed tests help you make sure version changes don’t slow things down. Twilio, the cloud communications platform, has a smart way of doing this:
-
They test how fast each API endpoint is normally.
-
After making changes, they test again to see if anything slowed down.
-
Twilio simulates real-world traffic from different parts of the world.
This helped Twilio keep their API up and running 99.95% of the time in 2023, even when they were making big changes.
Security Checks
New API versions can open up security holes if you’re not careful. Salesforce does a great job checking for these:
- They use automated tools to scan new API code for problems.
- Salesforce regularly tries to hack their own API to find weak spots.
- They use AI-powered tools to spot potential security issues.
These steps helped Salesforce cut down API-related security problems by 76% in 2023 compared to the year before.
Usage Tracking
It’s important to know which API versions people are actually using. This helps you decide what to update and what to get rid of. Google Cloud does this well:
- They track detailed info on how different API versions are being used.
- Google Cloud gives developers dashboards showing their API usage by version.
- They use this data to decide when to retire old versions and what to improve.
In 2023, this smart approach let Google Cloud trim down their API offerings. They cut the number of supported versions by 30% without causing much trouble for users.
Tools for API Versions
Managing API versions doesn’t have to be a headache. Let’s look at some tools that can make your life easier.
Code Version Tools
Git is the go-to for tracking API code changes. GitHub and GitLab are popular platforms that build on Git.
GitHub is a standout. It’s not just for version control - it’s a collaboration powerhouse. In 2023, GitHub saw a 35% jump in API-related repos. Developers are flocking to it for a reason.
API Gateways
API gateways are like traffic cops for your APIs. They handle versioning, security, and more.
Amazon API Gateway is a big player here. It plays nice with other AWS services and supports multiple API versions. The results speak for themselves: AWS users cut their API management work by 40% on average in 2023.
Kong Gateway is an open-source option gaining steam, especially for microservices. One user put it simply: “Kong turned our API deployment from a days-long slog to a hours-long sprint.”
Documentation Tools
Good docs are crucial. Here are two tools that can help:
Swagger UI is a classic for a reason. It works with OpenAPI Spec docs, making it a breeze to create and update API docs as you go.
ReadMe takes it a step further. It doesn’t just generate docs - it gives you the scoop on how people are using your API. One happy customer reported: “ReadMe slashed our support tickets by 30%. Clear, version-specific docs made all the difference.”
Testing Tools
You can’t skimp on testing, especially across API versions. These tools have got your back:
Postman is the Swiss Army knife of API testing. It lets you create test suites for different API versions, which is why 78% of developers in a 2023 survey said they use it.
SoapUI is another solid choice, especially if you’re dealing with SOAP APIs. It’s got some serious automation chops for testing across versions.
Conclusion
API versioning isn’t just a tech thing - it’s a must-have strategy for 2024 and beyond. Good versioning keeps your API stable, lets you innovate, and builds trust with your users.
Here’s what we’ve learned:
- Plan versioning from the start
Don’t wait. Think about versioning from day one. It’ll save you a ton of trouble later.
- Use semantic versioning
The major.minor.patch system is clear and easy to understand. It’s why big names like Stripe and GitHub use it.
- Keep old versions working
Amazon’s CTO Werner Vogels said it best: “APIs are forever.” Try to keep old versions running even as you add new stuff.
- Tell users about changes
Be clear about what’s new or different. Write good changelogs and guides. Give plenty of warning before you retire old versions. Twilio gave a six-month heads-up before shutting down an old SMS API version. That’s how it’s done.
- Support multiple versions
Let users upgrade when they’re ready. It keeps things smooth and your users happy.
- Make security a priority
Every version needs tight security. Salesforce used AI tools to spot potential issues, cutting API security problems by 76% in 2023.
- Test, test, test
Run lots of automated tests on all versions. Stripe runs over 10,000 tests for each update. They caught 37 potential problems before they went live.
- Use the right tools
From Git for version control to Amazon API Gateway, the right tools make versioning easier.