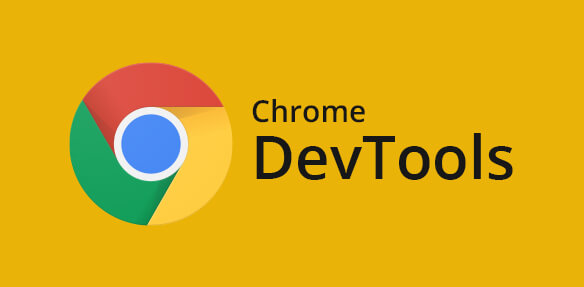
Debugging JavaScript in Chrome DevTools is easier than you think. Whether you’re fixing bugs, analyzing performance, or optimizing your code, Chrome DevTools provides powerful tools like breakpoints, source maps, and live expressions. Here’s what you need to know:
- Key Features: Debug JavaScript in real-time, inspect the DOM, track asynchronous call stacks, and analyze performance bottlenecks.
- Getting Started: Open DevTools with shortcuts (
CTRL+SHIFT+I
for Windows/Linux orCMD+OPT+I
for macOS) or right-click on a webpage and select “Inspect.” - Advanced Tools: Use conditional breakpoints, monitor events, and leverage the Network and Performance panels for deeper insights.
- Comparison: Chrome DevTools excels in debugging and performance analysis, while tools like Hoverify complement it with quick inspection and productivity-focused features.
For a seamless debugging experience, pair Chrome DevTools’ in-depth capabilities with complementary tools to streamline your workflow and fix issues faster.
Debugging JavaScript in Chrome DevTools
How to Start Using Chrome DevTools
Chrome DevTools offers a range of debugging tools directly in your browser. Whether you’re using Windows, macOS, or Linux, it’s simple to get started.
How to Open Chrome DevTools
You can open Chrome DevTools using these shortcuts: CTRL
+SHIFT
+I
for Windows/Linux or CMD
+OPT
+I
on macOS. Another way is to right-click any element on a webpage and choose “Inspect” [1] [6]. If you want direct access to the console, use CTRL
+SHIFT
+C
(Windows/Linux) or CMD
+OPT
+C
(macOS).
Adjusting DevTools and Activating Advanced Options
To customize your DevTools, click the three-dot menu in the top-right corner of the DevTools interface [2]. For debugging JavaScript, enable source maps by navigating to Settings > Preferences > Sources. This maps minified code back to its original files, making it easier to work with [5]. You can also turn on experimental features like live expressions, which let you track values in real time. Setting up workspaces allows you to save changes directly to your local files during debugging.
Once you’ve tailored DevTools to your needs, you’ll be equipped to dive into advanced debugging and improve your development workflow.
Key Debugging Techniques in Chrome DevTools
Chrome DevTools offers a range of tools to help developers quickly identify and resolve JavaScript issues. Here are some of the most useful debugging techniques to streamline your workflow.
Inspecting and Editing Elements
The Elements panel lets you examine and modify the DOM and CSS in real time. You can access it using Command + Option + C
on Mac or CTRL + Shift + C
on Windows/Linux [1]. Some key features include:
- Viewing and editing DOM elements and CSS styles live
- Running JavaScript directly on selected elements
- Debugging event listeners and DOM-related interactions
While the Elements panel focuses on the DOM, the Console is your go-to for JavaScript insights.
Using the Console for Debugging
The Console panel is perfect for quick troubleshooting and testing [3] [4]. Here are some handy debugging techniques:
console.log('Variable value:', variableName);
console.table(arrayOrObject);
You can also use debug()
to track function calls and monitorEvents()
to observe DOM events. These tools provide instant feedback and help you pinpoint issues.
For a deeper dive into your code, check out the Sources panel, which allows you to directly inspect and modify JavaScript files.
Debugging JavaScript Source Files
The Sources panel is a powerful tool for debugging JavaScript. It includes features like:
- Mapping local files to network resources for direct editing
- Debugging bundled or transpiled files using source maps
- Analyzing function execution with the Call Stack pane [1]
These capabilities make it easier to trace and resolve complex issues in your code.
Tracking Values with Live Expressions
Live expressions let you monitor variables in real time, offering another way to debug alongside breakpoints and console logs [1]. To set one up:
- Click the “Create Live Expression” button (eye icon)
- Enter your desired expression
- Watch as it updates in real time during code execution
For even more precision, pair live expressions with conditional breakpoints to focus on specific scenarios. You can also use the blackboxing feature to exclude third-party code from your debugging process, keeping your focus on what matters [1].
Advanced Tools for Debugging in Chrome DevTools
Once you’ve mastered the basics, Chrome DevTools offers tools to handle more complex debugging challenges. Here’s how you can make the most of these advanced features.
Using Breakpoints for Debugging
In the Sources panel, you can set conditional breakpoints to pause code execution only when certain conditions are met. Right-click a line number, select ‘Add conditional breakpoint,’ and define the condition. This is especially useful for diagnosing tricky issues in your code.
// Example of a conditional breakpoint
if (userCount > 1000) {
processUsers(); // Set breakpoint with condition: userCount === 1500
}
Debugging Network and Performance Issues
The Network panel is your go-to tool for analyzing API calls and resource loading. It helps you examine:
- Request headers and timing
- Resource loading patterns
- CORS errors
- Failed API calls
For performance-related issues, the Performance panel is invaluable. Use it to identify bottlenecks in CPU, memory, or network usage. Key metrics to monitor include:
Metric | What It Helps Diagnose |
---|---|
CPU Usage | Slow or inefficient scripts |
Memory Allocation | Potential memory leaks |
Network Activity | Inefficient resource loading |
Debugging Security Issues
The Security panel is designed to uncover vulnerabilities like certificate errors or mixed content warnings. Use it to:
- Verify certificate validation
- Check CORS configurations
- Identify security-related console errors
For a thorough approach, pair the Console and Security panels. Together, they provide a clearer picture of vulnerabilities and how to address them effectively.
Conclusion: Mastering JavaScript Debugging with Chrome DevTools
Key Takeaways
Chrome DevTools offers developers a powerful suite of tools for inspecting, debugging, and analyzing performance in real-time. By using features like source maps, you can debug your original code even when working with transpiled or minified JavaScript [5]. This is especially useful for working with modern frameworks and build tools.
What to Do Next
Here’s how you can build your debugging skills:
-
Start with the Basics: Use the Console panel for simple debugging tasks. Get comfortable with tools like
console.log()
, breakpoints, and the step-through debugger. Start with smaller projects to build confidence before tackling more complex applications [1] [3]. -
Explore Advanced Features: Once you’ve mastered the basics, dive into advanced tools. Learn how to use blackboxing to hide unnecessary scripts, performance profiling to fine-tune resource usage, and source maps to work with original code.
-
Streamline Your Workflow: Chrome DevTools is robust, but pairing it with tools like Hoverify can improve productivity. This combination lets you handle both in-depth debugging and everyday development tasks with ease.
Regular practice with debugging scenarios will not only help you fix issues faster but also guide you in writing cleaner, more efficient code from the start.
FAQs
Here are answers to some common questions about debugging JavaScript using Chrome DevTools.
How do I debug using the Console in Chrome?
To access the Console in Chrome DevTools, you can:
- Right-click on a webpage and choose “Inspect”.
- Go to Chrome’s menu, then More tools > Developer tools.
- Use keyboard shortcuts:
Ctrl + Shift + J
(Windows) orCmd + Option + J
(macOS).
The Console lets you run JavaScript commands, check errors, test code snippets, and observe variables in real time. It’s perfect for quick debugging, but for more detailed debugging, you’ll want to explore other DevTools features.
How can I debug JavaScript code in Chrome?
Debugging JavaScript in Chrome involves several key tools and methods:
-
Sources Panel
This is where you can map local files to network resources, debug transpiled code using source maps, and analyze function execution with call stacks. -
Breakpoints
Use conditional breakpoints to pause execution based on specific criteria. You can also insert thedebugger
statement directly into your code or combine breakpoints with watch expressions for deeper analysis. -
Console Methods
Tools likeconsole.table()
help visualize data in a structured format. UsemonitorEvents()
to track DOM activity ordebug()
to pause on specific function calls. -
Performance Tools
Check the Network tab for API calls and requests, monitor Core Web Vitals, and track memory usage to identify potential leaks.
“Best practices include using breakpoints strategically, leveraging the Console for quick testing and logging, and utilizing the Sources tab for in-depth debugging of JavaScript source files” [1] [6].