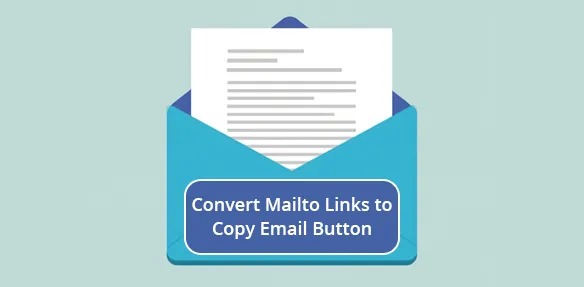
Have you ever clicked a mailto link accidentally and watched your email client spring to life uninvited? While mailto links serve a purpose, many users prefer to simply copy email addresses instead of launching their email application. This tutorial will show you how to transform all mailto links on any website to copy the email address to your clipboard with a single click.
We’ll use Hoverify’s “Custom Code” feature to inject our JavaScript code that handles this transformation. Once set up, it will automatically work on any page you visit, making email address handling much more convenient.
How to Convert Mailto Links to Copy Functionality
Step 1: Create a New Script
- Open the Hoverify popup and click on the “Debug” tool.
- Navigate to “Custom Code” in the tools panel.
- Click “New” to create a fresh script.
Step 2: Configure the Host
- In the host field, enter ”*” (asterisk) to apply this script to all websites.
- Alternatively, specify particular domains if you only want this behavior on certain sites.
- Click “Create” to proceed to the editor.
Step 3: Add the Code
Here’s the JavaScript code that transforms mailto links:
<script>
const mailtoLinks = document.querySelectorAll('a[href^="mailto:"]');
mailtoLinks.forEach(link => {
const email = link.href.replace('mailto:', '').split('?')[0];
link.dataset.email = email;
link.addEventListener('click', function(e) {
e.preventDefault();
// Copy email to clipboard
navigator.clipboard.writeText(email);
try {
// Show feedback to user
const originalText = link.textContent;
link.textContent = 'Email copied!';
// Reset text after 2 seconds
setTimeout(() => {
link.textContent = originalText;
}, 2000);
}
catch (err) {
console.error('Failed to copy email:', err);
alert('Could not copy email address. Please try again.');
}
});
// Add a title attribute for hover tooltip
link.title = `Click to copy: ${email}`;
});
</script>
Step 4: Save Your Script
- Press CTRL + S or click the “Save” button.
- Name your script something memorable like “Mailto to Copy”.
- Click “Save” to finalize.
Step 5: Test the Functionality
- Visit any webpage with mailto links.
- Hover over a mailto link to see the new tooltip.
- Click the link: instead of opening your email client, it should copy the address.
- Look for the visual feedback showing the email was copied.
How the Script Works
Let’s break down what this code does:
- The script is injected when the page loads and finds all links that start with “mailto:” using
querySelectorAll
. - For each mailto link, it extracts the email address from the href attribute, removing any additional parameters after the ”?” symbol.
- Stores the clean email address in the link’s dataset for easy access.
- Adds a click handler that:
- Prevents the default mailto behavior.
- Uses the Clipboard API to copy the email address.
- Provides visual feedback by temporarily changing the link text.
- Includes error handling if the clipboard operation fails.
- Adds a helpful tooltip showing what will happen on click.
Customization Options
You can enhance the main script with these modifications:
- Change the feedback message by modifying the try block:
// Within the try block of the main script
link.textContent = 'Copied to clipboard!'; // Change to your preferred message
- Adjust the feedback duration by updating the setTimeout:
// Within the try block of the main script
setTimeout(() => {
link.textContent = originalText;
}, 3000); // Change from 2000 to your preferred duration
- Add custom styling by including this in your script:
const style = document.createElement('style');
style.textContent = `
a[href^="mailto:"] {
cursor: copy;
/* Add any other styles you want */
}
`;
document.head.appendChild(style);
Troubleshooting
If you encounter any issues:
- Make sure your browser supports the Clipboard API (most modern browsers do).
- Check the browser’s console for any error messages.
- Verify that the script is running on your intended pages by checking for the hover tooltips.
- Ensure no other scripts are interfering with mailto links by temporarily disabling other extensions.
This solution provides a more user-friendly approach to handling email addresses on websites, giving users the flexibility to copy addresses without launching their email client. The visual feedback and hover tooltips make the functionality clear and intuitive.
Remember that this script modifies the default behavior of mailto links. Consider using specific domain patterns in Hoverify’s host field rather than ”*” if you only want this behavior on certain websites.