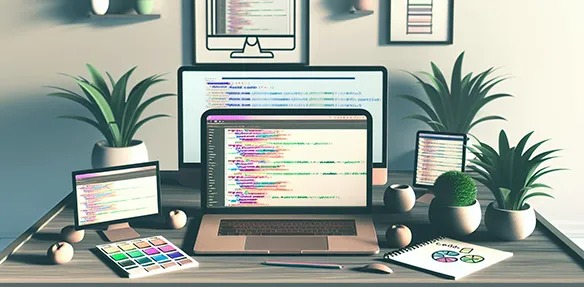
CSS browser compatibility can be a headache, but it’s crucial for creating websites that work well across different platforms. Here’s what you need to know:
- Different browsers interpret CSS differently, causing inconsistent website appearance and functionality
- Main causes: varying rendering engines, CSS support levels, and default styles
- Key strategies to address compatibility issues:
- Use CSS resets or normalization
- Add vendor prefixes
- Create fallbacks for unsupported features
- Test on multiple browsers and devices
Quick comparison of major browser rendering engines:
Engine | Used by | Key Feature |
---|---|---|
Blink | Chrome, Opera, Edge | LayoutNG for faster, flexible layouts |
Gecko | Firefox | Parallel CSS parsing with Stylo |
WebKit | Safari, iOS browsers | Traditional WebCore approach |
Remember: Cross-browser testing is essential. Use tools like BrowserStack or manual testing to ensure your site works well everywhere.
By understanding these differences and implementing the right strategies, you can create websites that look and function consistently across all major browsers.
Browser rendering engines
Browser rendering engines are the heart of web browsers. They interpret and display web content. Let’s look at the main ones and how they affect CSS compatibility.
Major rendering engines
Three engines power most modern browsers:
- Blink: Google’s engine. Used in Chrome, Opera, Brave, and Edge.
- Gecko: Mozilla’s engine. Powers Firefox.
- WebKit: Apple’s engine. Used in Safari and iOS browsers.
Each engine handles CSS differently. This can cause compatibility issues. For example, Firefox and Safari don’t support the animation-timeline
CSS property. This means animations might not work the same across all browsers.
How engine differences affect CSS
These differences can lead to:
- Layout problems
- Some CSS properties not working in certain browsers
- Performance differences
Here’s a quick look at how each engine handles layouts:
Engine | Layout Approach | Key Feature |
---|---|---|
Blink | LayoutNG | Faster, flexible layouts |
WebKit | WebCore | Traditional approach |
Gecko | Stylo | Parallel CSS parsing |
Blink’s LayoutNG can handle complex, dynamic layouts better than others. This might cause display issues in other browsers.
That’s why cross-browser testing is so important. Chris, who runs an Elderly Care website, learned this the hard way:
“I used CSS text-orientation that didn’t work in IE11. After testing, I fixed the bugs and my site now works for more users.”
Understanding these differences helps developers write better, more compatible CSS. It’s key to creating websites that work well across all browsers.
Common CSS compatibility problems
CSS can be tricky across browsers. Here are the main issues developers face:
Layout issues
Browsers can interpret CSS layouts differently. For example:
- Flexbox images often have size problems
- Animated grids work in Firefox but break in Chrome and Safari
To fix this:
-
Use a CSS reset
-
Test on multiple browsers
-
Use feature detection
Font rendering problems
Fonts can look different across browsers:
OS | Rendering Tech | Result |
---|---|---|
Windows | GDI/DirectWrite | Varies |
Mac OS | Quartz | More consistent |
To improve:
-
Use
font-weight: normal
andfont-style: normal
in@font-face
-
Use well-hinted TrueType fonts
-
Try
-webkit-text-stroke
or text-shadow
Box model differences
Browsers can interpret the CSS box model differently.
Fix it by:
-
Using a CSS reset
-
Applying
box-sizing: border-box
CSS property support issues
Some CSS properties don’t work in all browsers, especially older ones.
Browser | CSS Support |
---|---|
Chrome (106+) | Full |
Edge (107+) | Full |
Safari (15.6+) | Full |
Firefox (106+) | Full |
Opera (92+) | Full |
For limited support:
-
Use vendor prefixes
-
Implement fallbacks
-
Test on BrowserStack
CSS reset and normalization
CSS reset and normalization help create consistent styling across browsers. Here’s what you need to know:
Why use CSS resets?
CSS resets wipe out default browser styles. This:
- Cuts down browser differences
- Gives you more style control
- Makes layout math easier
Popular CSS reset options
- Eric Meyer’s Reset CSS
Zeros out default CSS properties or sets them to basic values.
- HTML5 Reset Stylesheet
Richard Clark’s update of Meyer’s reset for HTML5.
- CSS Mini Reset
A tiny reset with just four rules for common HTML elements.
- Josh Comeau’s Custom CSS Reset
A modern take that improves user and dev experience.
Here’s a taste of Josh’s reset:
*, *::before, *::after { box-sizing: border-box; }
* { margin: 0; }
body { line-height: 1.5; -webkit-font-smoothing: antialiased; }
img, picture, video, canvas, svg { display: block; max-width: 100%; }
input, button, textarea, select { font: inherit; }
p, h1, h2, h3, h4, h5, h6 { overflow-wrap: break-word; }
#root, #__next { isolation: isolate; }
How to use CSS normalization
Normalization standardizes styles while keeping useful defaults. Here’s how:
-
Pick a library like Normalize.css or sanitize.css.
-
Add it to your project. For Normalize.css:
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/normalize/8.0.1/normalize.min.css">
- Put your custom styles after the normalization styles.
Approach | Purpose | Example |
---|---|---|
CSS Reset | Nuke all default styles | Eric Meyer’s Reset CSS |
CSS Normalization | Make styles consistent across browsers | Normalize.css |
Vendor prefixes
Vendor prefixes help with CSS browser compatibility. They let you use new CSS features before all browsers fully support them.
What are vendor prefixes?
Vendor prefixes are keywords added to CSS properties. They tell browsers to use their own versions of a CSS feature. Here are some common ones:
Prefix | Browser |
---|---|
-webkit- | Chrome, Safari, newer Opera |
-moz- | Firefox |
-ms- | Internet Explorer and Edge |
-o- | Old Opera versions |
How to use them
To use vendor prefixes:
- Add prefixed versions of a property
- Include the standard version last
Like this:
.box {
-webkit-border-radius: 10px;
-moz-border-radius: 10px;
border-radius: 10px;
}
This way, the standard property kicks in once browsers support it.
Tools to make it easier
Adding prefixes by hand? That’s a pain. Here are some tools to help:
- Autoprefixer: A PostCSS plugin that adds prefixes based on current browser data.
- -prefix-free: A JavaScript library that adds prefixes on the client-side.
- Prefixr: Parses your CSS and adds missing prefixes before you deploy.
- CSS preprocessor mixins: Sass and Less have mixins to handle prefixes.
“Autoprefixer has been recommended by Google and used by Twitter and Alibaba Group.” - Autoprefixer documentation
These tools can save you time and headaches with your CSS.
Feature detection and fallbacks
Feature detection is crucial for making websites work across different browsers. It’s all about managing CSS features that some browsers might not support.
Using feature detection libraries
Modernizr is a go-to JavaScript library for feature detection. It checks if a browser can handle specific CSS features and adds classes to the HTML tag.
Here’s a quick example:
.no-cssgradients .header {
background: url("images/gradient.png");
}
.cssgradients .header {
background-image: linear-gradient(blue, purple);
}
This lets you serve up different styles based on what the browser can handle.
Creating fallbacks
CSS has a built-in way to check for feature support: the @supports
rule. It works in most modern browsers, but Internet Explorer isn’t a fan.
Here’s how it looks:
@supports (display: grid) {
.container {
display: grid;
}
}
@supports not (display: grid) {
.container {
display: flex;
}
}
This code uses CSS Grid if it’s supported, and falls back to flexbox if it’s not.
For older browsers, you can use CSS’s fault tolerance. Browsers ignore CSS they don’t understand, so you can layer properties:
.overlay {
background: grey;
background: rgba(0, 0, 0, 0.4);
border: 1px solid grey;
border: 1px solid rgba(0, 0, 0, 0.4);
}
Older browsers use the solid color, while newer ones go for the rgba value.
Progressive enhancement
Progressive enhancement is about starting simple and adding fancy features for browsers that can handle them.
Start with a basic layout, then jazz it up for modern browsers:
.container {
/* Basic layout */
display: block;
}
@supports (display: flex) {
.container {
display: flex;
justify-content: space-between;
}
}
This way, your site works for everyone, but users with modern browsers get a little extra.
“The core concept is not to ask ‘What browser is this?’ It’s to ask ‘Does your browser support the feature I want to use?‘” - Rob Larson, The Uncertain Web: Web Development in a Changing Landscape (2014).
Browser-specific CSS fixes
Building websites? You’ll hit CSS issues in certain browsers. Here’s how to fix them:
Internet Explorer (IE) fixes
IE’s been a pain for developers. Microsoft killed it, but some still use old versions.
For IE9 and below:
<!--[if IE]>
<style>
/* IE-specific styles */
</style>
<![endif]-->
For IE10 and IE11:
@media all and (-ms-high-contrast: none), (-ms-high-contrast: active) {
/* IE10+ styles */
}
IE hacks
Hack | Target | Example |
---|---|---|
* html | IE6 and below | * html .box { color: red; } |
*+html | IE7 | *+html .box { color: blue; } |
\9 | IE8 | .box { color: green\9; } |
WebKit browser fixes
WebKit powers Chrome and Safari. They’re usually good with CSS, but sometimes need tweaks.
For Safari, try:
.button {
-webkit-appearance: none;
appearance: none;
}
This strips default styling on form elements.
For older WebKit border-radius:
.circle {
-webkit-border-radius: 50%;
border-radius: 50%;
}
Firefox fixes
Firefox is solid with CSS, but occasionally needs help. Here’s a trick using Mozilla bindings:
- Make an XML file (
firefox-fix.xml
):
<?xml version="1.0"?>
<bindings xmlns="http://www.mozilla.org/xbl">
<binding id="firefox-css">
<implementation>
<constructor>
<![CDATA[
var link = document.createElement("link");
link.setAttribute("rel", "stylesheet");
link.setAttribute("href", "firefox.css");
document.head.appendChild(link);
]]>
</constructor>
</implementation>
</binding>
</bindings>
- Use the binding in HTML:
<style>
body {
-moz-binding: url(firefox-fix.xml#firefox-css);
}
</style>
- Create
firefox.css
:
/* Firefox-specific styles */
h1 { color: red; }
This loads Firefox styles only when needed.
Cross-browser testing
Cross-browser testing is key for web developers. It ensures your CSS works across browsers and devices. Here’s how to do it:
Manual testing
The classic approach: test on real browsers. It’s slow but gives you a true user perspective. Quick checklist:
- Open your site on Chrome, Firefox, Safari, and Edge
- Check layout, fonts, and colors
- Test forms and interactive elements
- View your site on different screen sizes
Pro tip: Use browser dev tools to mimic mobile devices.
Automated tools
Automated tools save time by testing on multiple browsers at once. Some options:
Tool | Feature | Price |
---|---|---|
BrowserStack | Real devices | From $29/month |
LambdaTest | 3000+ combos | From $25/month |
Sauce Labs | CI/CD ready | Custom |
These tools can screenshot, run tests, and record your site in action.
Older browser testing
Don’t ignore older browsers. Some users still have them. To test:
-
Set up VMs with old OS versions
-
Install older browsers on these VMs
-
Run your site and look for issues
“Real device cloud testing beats old web browser emulators. Emulators can’t fully replicate real browser features and flaws.” - BrowserStack docs
Your site doesn’t need to be identical everywhere. But it should work and not break.
Writing compatible CSS
Want your CSS to work smoothly across browsers? Here’s how:
Clean and modular CSS
Break your CSS into logical chunks:
/styles
/base
reset.css
typography.css
/components
button.css
navbar.css
/layout
grid.css
/utilities
mixins.css
This structure makes your code easier to manage.
Use clear class names:
/* Not great */
.bx { ... }
/* Much better */
.product-box { ... }
Clear names help you (and others) understand the code quickly.
Ditch browser-specific hacks
Stick to standard CSS when you can. Use feature detection instead of hacks:
if ('flexGap' in document.body.style) {
// Browser supports gap in flexbox
} else {
// Use fallback
}
This works better than targeting specific browsers, which can break with updates.
Document special code
Add comments for any browser-specific tweaks:
/* Fix for IE11 flexbox bug */
.flex-container {
display: flex;
flex-direction: column;
}
These comments explain why certain code exists.
Don’t forget:
- Use a CSS reset or Normalize.css for a consistent base.
- Test on multiple browsers and devices.
- Use Autoprefixer to add vendor prefixes automatically.
Using new CSS features safely
Want to use modern CSS but worried about browser support? Here’s how to do it right:
CSS Grid with fallbacks
CSS Grid is awesome, but older browsers don’t get it. Here’s the fix:
.container {
display: flex;
flex-wrap: wrap;
}
@supports (display: grid) {
.container {
display: grid;
grid-template-columns: repeat(3, 1fr);
}
}
This gives you a flex layout for old browsers and Grid for the new ones.
Flexbox compatibility
Flexbox is pretty well-supported, but there are still some quirks:
.flex-container {
display: -webkit-box;
display: -webkit-flex;
display: -ms-flexbox;
display: flex;
}
.flex-item {
-webkit-box-flex: 0;
-webkit-flex: 0 1 auto;
-ms-flex: 0 1 auto;
flex: 0 1 auto;
}
Use vendor prefixes and explicit flex values to avoid issues in older browsers.
Don’t forget to test your layouts in different browsers, especially IE and old Safari versions.
CSS variables with fallbacks
CSS variables are cool, but not all browsers support them. Here’s how to use them safely:
.button {
background-color: #007bff;
background-color: var(--primary-color, #007bff);
}
This sets a fallback color for browsers that don’t support CSS variables.
For broader support, you can use feature detection:
@supports (--css: variables) {
:root {
--primary-color: #007bff;
}
.button {
background-color: var(--primary-color);
}
}
If you REALLY need to support ancient browsers, consider using a CSS variables polyfill.
Debugging CSS across browsers
Debugging CSS in different browsers can be a pain. Here’s how to do it:
Use browser dev tools
Every major browser has built-in dev tools. Open them with F12 or Ctrl+Shift+I (Cmd+Option+I on Mac).
These tools are your best friends for CSS debugging. You can:
- Toggle CSS rules
- Edit properties on the fly
- Check element box models
- See computed styles
Spot browser-specific issues
To find browser-specific problems:
- Test your site in multiple browsers
- Use tools like BrowserStack
- Check CSS support on caniuse.com
When you find an issue, isolate it with browser-specific CSS:
@supports (-webkit-appearance:none) {
/* WebKit CSS */
}
@-moz-document url-prefix() {
/* Firefox CSS */
}
Compare browser outputs
To see how browsers render your CSS:
- Take screenshots in each browser
- Use a visual diff tool
- Look at layout, fonts, and spacing
Browser | Common Issues | Debug Method |
---|---|---|
Chrome | Flexbox bugs | Use Flexbox inspector |
Firefox | Font rendering | Check font-smoothing |
Safari | Grid layout | Test with Tech Preview |
IE/Edge | CSS3 support | Use compatibility mode |
Don’t aim for pixel-perfect matches. Focus on consistent rendering across browsers.
“CSS is designed to not lose content, to not cause harm. In order to not cause harm, we show what overflows by default.” - Miriam Suzanne, CSS Expert
This keeps your content visible while you fix layout issues.
Performance considerations
Balancing CSS browser compatibility with performance is key. Here’s how to optimize CSS across browsers without slowing down your site.
Compatibility vs. performance
Adding extra CSS for compatibility can hurt load times. To avoid this:
- Use feature detection, not browser-specific hacks
- Apply progressive enhancement
- Test thoroughly to avoid new performance issues
Optimizing CSS for browsers
Deliver CSS efficiently across browsers:
- Minify and compress CSS
Cut file size by removing unnecessary characters. Use tools like Google’s PageSpeed Insights to find minification opportunities.
- Use HTTP/2 push
Send CSS files to the browser before they’re requested, speeding up rendering.
- Implement critical CSS
Inline above-the-fold CSS in the HTML to reduce render-blocking and improve initial load times.
Technique | Description | Impact |
---|---|---|
Minification | Remove whitespace and comments | 20-25% file size reduction |
Compression | Use GZIP or Brotli | Up to 70% transfer size reduction |
Critical CSS | Inline essential styles | Better First Contentful Paint |
Reducing CSS bloat
Trim unnecessary CSS:
- Use DevTools CSS Coverage to find unused styles
- Organize CSS into modules
- Regularly audit and remove old compatibility fixes
“Google cares about performance. Does your site load quick? Well, not if you load a large CSS file designated for your entire site and not for the current page.” - Jonathan “JD” Danylko
Long-term compatibility
Future-proofing CSS
Want your CSS to stay fresh? Here’s how:
- Use feature queries
Check if a browser can handle new CSS tricks:
@supports (display: grid) {
.container {
display: grid;
}
}
This way, you’re covered even if some browsers can’t keep up.
- Go mobile-first
Start simple, then jazz it up for bigger screens. Your site will work everywhere.
- Think logically
Use direction-neutral properties like margin-inline-start
. It’s a lifesaver for different languages and layouts.
Keep tabs on browsers
Stay in the loop:
- Read browser blogs
- Check caniuse.com for feature support
- Hang out in web dev communities
Maintenance game plan
Keep your CSS ship-shape:
What to do | How often | Why bother |
---|---|---|
Review code | Monthly | Spot old-school stuff |
Test browsers | Quarterly | Catch weird issues |
Check speed | Twice a year | Make CSS faster |
Clean up | When needed | Tidy your code |
Pro tip: Use stylelint to catch mistakes between manual checks.
“We’ll never have a world where everyone’s browser is the same. Just like we’ll never have identical screens and resolutions.”
This quote nails why we need to keep our CSS in check. Different browsers, different devices - it’s a jungle out there!
Conclusion
CSS browser compatibility is crucial for web development. Here’s how to tackle it:
- Use CSS resets
Normalize.css or Reset CSS create a consistent styling baseline across browsers.
- Leverage vendor prefixes
Apply -webkit-, -moz-, -ms-, and -o- prefixes for non-standard CSS properties. Autoprefixer can manage these automatically.
- Implement feature detection
Use JavaScript libraries like Modernizr to check browser support for specific CSS features.
- Adopt progressive enhancement
Start with a basic experience that works everywhere, then add advanced features for capable browsers.
Stay Updated
The web dev landscape is always changing. To keep up:
- Read browser release notes and developer blogs
- Check caniuse.com for feature support
- Join web development communities and forums
Balance Compatibility and New Features
Aspect | Approach |
---|---|
Browser support | Focus on high market share browsers |
Testing | Use BrowserStack or Sauce Labs for cross-browser testing |
Performance | Optimize CSS for speed while maintaining compatibility |
Maintenance | Regularly update and test CSS for new compatibility issues |
Cross-browser compatibility is ongoing. As Akshay Kothari, CPO of Notion, said after their Product Hunt launch:
“The web moves fast. What works today might not work tomorrow. We have to stay vigilant and keep testing to ensure our product looks and functions great across all browsers.”
FAQs
Is CSS compatible with all browsers?
Nope, CSS isn’t a one-size-fits-all deal for browsers. Different browsers can be picky about how they handle CSS properties and values. This means your webpage might look great in Chrome but wonky in Safari.
Take CSS Filters, for example. Some browsers might ignore certain filter effects entirely, while others might interpret them in their own unique way. The result? Your carefully crafted design could look different depending on the user’s browser choice.
How do I check if CSS is supported by browser?
Want to know if a browser can handle a specific CSS feature? Enter the @supports
rule. It’s like a little test you can run in your CSS. Here’s how it works:
@supports (display: grid) {
.container {
display: grid;
}
}
This snippet says, “Hey browser, if you can handle grid layouts, go ahead and use it for the container class.” If the browser can’t, it’ll just skip over this part.
Which browser supports CSS?
Most modern browsers play nice with CSS, but some are better team players than others. Here’s a quick rundown of browsers with solid CSS support:
Browser | Version |
---|---|
Chrome | 106+ |
Edge | 107+ |
Safari | 15.6+ |
Firefox | 106+ |
Opera | 92+ |
But here’s the catch: while these browsers support most CSS features, they might stumble on newer or experimental stuff. So, always double-check support for specific features when you’re aiming for a design that works everywhere.