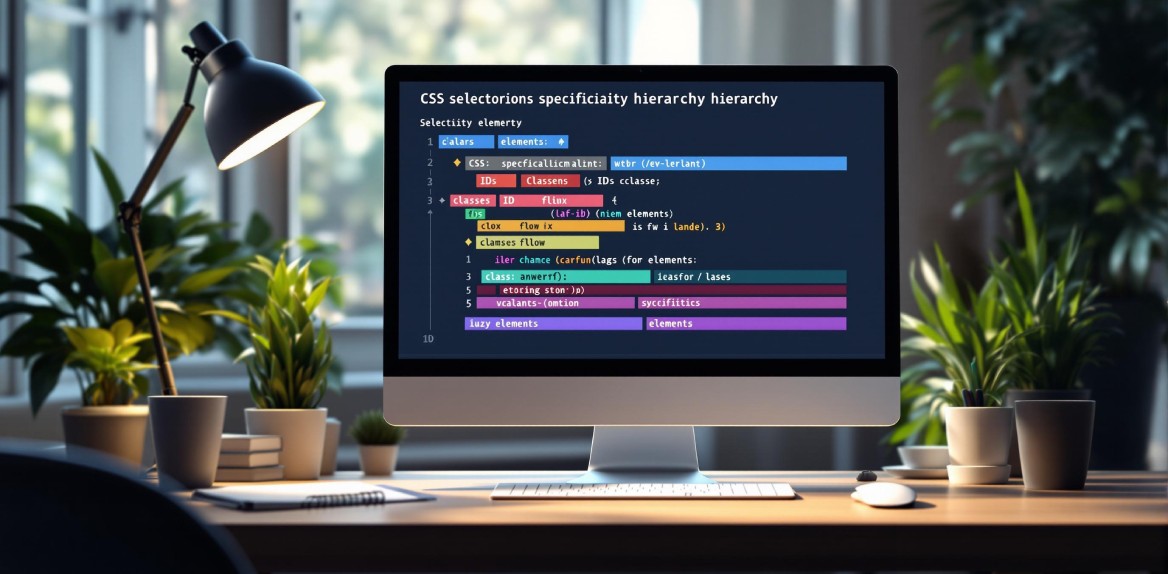
CSS specificity determines which styles apply when multiple CSS rules target the same element. Here’s what you need to know:
- Specificity Hierarchy: Inline styles rank highest, followed by ID selectors, class/attribute/pseudo-class selectors, and finally element/pseudo-element selectors.
- Specificity Scoring: Browsers use a four-part numerical system (0,0,0,0) to calculate specificity:
- Inline styles: 1,0,0,0
- ID selectors: 0,1,0,0
- Classes/attributes/pseudo-classes: 0,0,1,0
- Elements/pseudo-elements: 0,0,0,1
- Special Cases: Some selectors like
:not()
and:where()
have unique specificity rules.!important
overrides specificity but should be used sparingly. - Best Practices: Use classes for most styling, avoid overly complex selectors, and minimize the use of IDs and
!important
.
To debug specificity issues, tools like Hoverify can help you inspect and calculate specificity scores in real-time.
Quick Tip: Write clean, modular CSS to avoid conflicts and ensure styles behave predictably.
Specificity Rules and Rankings
Understanding how browsers rank CSS selectors is key to creating styles that are both predictable and easy to manage.
Selector Priority Levels
CSS specificity is ranked using a four-part numerical system (0,0,0,0). Each part corresponds to a different type of selector, ranked from highest to lowest priority:
Position | Selector Type | Example | Specificity Value |
---|---|---|---|
1st digit | Inline styles | style="color: red" | 1,0,0,0 |
2nd digit | ID selectors | #header | 0,1,0,0 |
3rd digit | Classes, attributes, pseudo-classes | .nav , [type="text"] , :hover | 0,0,1,0 |
4th digit | Elements, pseudo-elements | div , ::before | 0,0,0,1 |
When multiple selectors apply to the same element, browsers add up the values in each position. For example:
#nav .list-item:hover {
/* Specificity: 0,1,2,0 */
}
Special Selectors
Some selectors and combinators follow unique rules for specificity:
- Universal Selector (
*
): Doesn’t add any specificity. - Combinators (
+
,>
,~
): Don’t contribute to specificity scores. :not()
Pseudo-class: Only the specificity of its argument counts.:where()
Pseudo-class: Always has a specificity of zero.:is()
Pseudo-class: Uses the highest specificity among its arguments.
Keep in mind that !important
overrides specificity but should be used sparingly. If multiple !important
rules conflict, the usual specificity rules still apply to decide the winner.
Tools like Hoverify’s CSS inspector can help you quickly check the specificity of any selector, making it easier to understand which styles are applied or overridden.
Next, we’ll dive into practical examples to show you how to calculate specificity effectively.
Specificity Calculations
Here’s how you can calculate specificity scores for your CSS selectors effectively.
Basic Calculation Method
To calculate specificity, break down your selector and count each type. For example:
nav#main-nav .dropdown-menu li:hover > a {
color: blue;
}
Here’s the breakdown:
- Element selectors:
nav
,li
,a
(3 total) - ID selectors:
#main-nav
(1 total) - Classes/pseudo-classes:
.dropdown-menu
,:hover
(2 total)
Now map these counts to their specificity positions:
- Inline styles: 0
- IDs: 1
- Classes/pseudo-classes: 2
- Elements: 3
Final specificity score: 0,1,2,3
Calculation Tools
Tools like Hoverify’s CSS inspector make calculating specificity easier. As you hover over elements, it shows real-time specificity scores, helping you debug why certain styles are applied or overridden. This tool also highlights contributing selectors, displays the full specificity hierarchy, and allows you to test combinations directly in the interface.
Practice Examples
Here are some common selectors with their specificity breakdowns:
Selector | Breakdown | Specificity Score |
---|---|---|
#header .nav-item:hover | 1 ID, 1 class, 1 pseudo-class | 0,1,2,0 |
div.container p strong | 3 elements, 1 class | 0,0,1,3 |
[type="text"].input-field:focus | 1 attribute, 1 class, 1 pseudo-class | 0,0,3,0 |
main article p::first-line | 3 elements, 1 pseudo-element | 0,0,0,4 |
Specificity adds up when combining selectors. For instance:
.sidebar article.featured p strong {
/* Specificity: 0,0,2,3 */
/* (.sidebar = 0,0,1,0) */
/* (article.featured = 0,0,1,1) */
/* (p = 0,0,0,1) */
/* (strong = 0,0,0,1) */
}
Understanding these calculations helps you resolve conflicts faster and create cleaner, more maintainable CSS. Tools like Hoverify can streamline the process, making it easier to identify and fix specificity issues.
Fixing Specificity Issues
Let’s dive into how you can tackle CSS specificity problems effectively, building on the earlier explanation of how specificity is calculated.
Common Problems
Specificity issues in CSS often show up in these ways:
- Styles not applying: Higher specificity rules block new styles.
- Cascade conflicts: Multiple rules target the same element but with differing values.
- Overly complex selector chains: Hard to read and maintain.
- Overuse of
!important
: Used excessively to force styles. - Framework conflicts: Third-party CSS frameworks clash with your custom styles.
Here’s an example of a typical specificity conflict:
/* Framework CSS */
.btn-primary {
background-color: blue;
}
/* Your custom CSS */
.button {
background-color: green; /* Won’t apply because of lower specificity */
}
Debug Methods
When debugging specificity conflicts, tools like Hoverify’s Inspector can be incredibly helpful. Here’s how:
-
Real-Time Inspection
View which CSS rules are applied or overridden, along with the entire specificity hierarchy for any element. -
Visual Debugging
- Toggle styles on and off to pinpoint conflicts.
- Edit CSS live to test fixes instantly.
- Hide elements to check how styles are inherited.
- Inspect pseudo-classes without needing to trigger them manually.
Once you’ve identified the conflicts, you can address them by improving your CSS practices.
Writing Better CSS
Here are some tips to write CSS that avoids common specificity pitfalls:
Selector Strategy
- Stick to using classes as your main selector type.
- Use ID selectors sparingly - ideally, only one per component.
- Avoid styling directly with element selectors (reserve them for resets).
- Keep selector chains short, ideally 2-3 levels deep.
Naming Conventions
Instead of writing overly specific selectors, simplify them:
/* Avoid this */
header nav ul li a.nav-link {
color: blue;
}
/* Use this */
.nav-link {
color: blue;
}
Component-Based Approach
Adopting a modular, component-based approach can simplify your CSS and reduce conflicts:
/* Modular component styling */
.card {
background: white;
}
.card__title {
font-size: 1.25rem;
}
.card--featured {
border: 2px solid gold;
}
This approach ensures your styles are easier to manage, more predictable, and less prone to specificity issues.
Conclusion
Main Points
Understanding CSS specificity is essential for managing stylesheets effectively. Here are the key takeaways:
- Specificity Hierarchy: Styles are applied based on a clear order - inline styles rank highest, followed by IDs, classes, and then element selectors.
- Calculation Method: Each type of selector contributes a specific weight to the overall specificity score.
- Best Practices: Use classes instead of IDs, keep selectors concise, and reserve
!important
for rare, critical cases. - Component-Based Approach: Modular CSS helps reduce conflicts and makes styles easier to maintain.
Using Hoverify for CSS
Hoverify is a tool that simplifies CSS debugging by combining real-time inspection with advanced features, making it easier to apply specificity principles.
“Found an incredibly useful web design / development tool called Hoverify. Allows you to inspect elements on any site, copy styles, show grids, check on different viewports, grab asset lists, hide elements, and a whole lot more.” [1]
Hoverify helps manage CSS specificity with features like:
- Real-Time Inspection: Quickly analyze styles and their specificity.
- Visual Style Editor: Make live CSS changes and test them instantly.
- Pseudo-Class Inspector: Easily review element states like
:hover
or:focus
. - Box Model Visualization: Clearly see layout properties such as margins, padding, and borders.
“As a website developer, this plugin has so many handy features. One of my favorites is the ability to quickly take full page screenshots very easily, and the inspector tool and color selectors I use very often. There are lots of other different extensions or browser functions that offer these, but I love that everything is all in one place and consistent across different browsers and devices. Highly recommend.” [1]