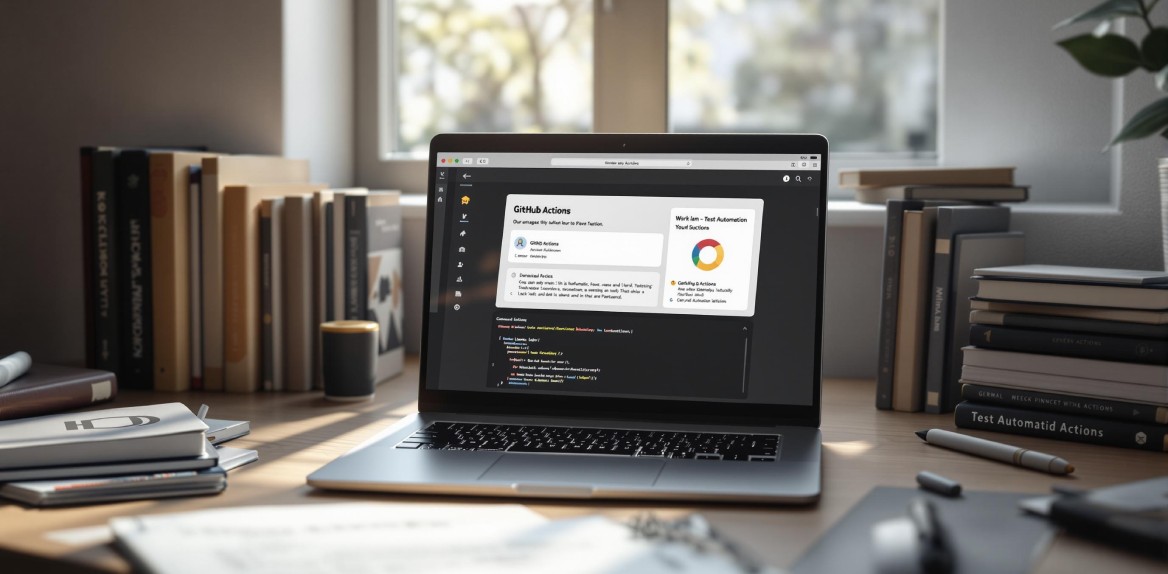
GitHub Actions makes test automation easier by integrating it directly into your GitHub repository. Here’s how it helps:
- Automates Testing Workflows: Runs tests automatically on code changes, pull requests, or scheduled events.
- Saves Time: Reduces manual testing efforts by up to 80%.
- Supports Multi-Environment Testing: Tests across different platforms, operating systems, and configurations.
- Prebuilt Tools: Access 10,000+ actions for popular frameworks like Jest, Cypress, and Pytest.
- Matrix Builds: Run tests simultaneously on multiple operating systems, Node.js versions, or databases.
- Faster Testing: Speeds up workflows with caching, parallel execution, and artifact storage.
Quick Overview:
Feature | Benefit |
---|---|
Built-in Integration | Works directly within GitHub |
Prebuilt Actions | Saves time with ready-to-use tools |
Multi-OS Support | Ensures cross-platform compatibility |
Caching & Parallelism | Reduces test execution times |
With GitHub Actions, you can create efficient, automated testing pipelines that ensure code quality across all environments.
Getting Started with GitHub Actions
This section walks you through setting up your first testing workflow.
Writing the Workflow File
To begin, create a file named .github/workflows/test.yml
to define your test automation workflow:
name: Run Tests
on: [push, pull_request]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Set up Node.js
uses: actions/setup-node@v3
with:
node-version: '18.x'
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
This file outlines the steps GitHub Actions will follow to run your tests. It also specifies the events that will trigger the workflow.
Setting Up Triggers
GitHub Actions allows you to run workflows based on specific events. Here are some common triggers:
Trigger Event | Purpose | Example Configuration |
---|---|---|
Push | Run tests on code commits | on: push |
Pull Request | Validate changes before merging | on: pull_request |
Schedule | Run tests at regular intervals | on: schedule: - cron: '0 0 * * *' |
Workflow Dispatch | Trigger tests manually | on: workflow_dispatch |
You can combine multiple triggers to match your team’s workflow. For instance:
on:
push:
branches: [ main, develop ]
pull_request:
branches: [ main ]
This configuration ensures tests run on pushes to main
and develop
branches and for pull requests targeting main
.
Selecting a Runner
The runner determines the environment where your tests will execute. GitHub Actions provides several options:
- Ubuntu Linux: Popular for web apps and Node.js projects.
- Windows: Ideal for .NET or Windows-specific testing.
- macOS: Best for iOS and macOS applications.
For cross-platform testing, you can use a matrix configuration to run tests on multiple operating systems:
jobs:
test:
strategy:
matrix:
os: [ubuntu-latest, windows-latest, macos-latest]
runs-on: ${{ matrix.os }}
This approach ensures your tests work consistently across different platforms, helping you catch any OS-specific issues early in the development process.
Adding Testing Tools to GitHub Actions
Once your basic test workflow is in place, you can enhance it by incorporating specialized testing tools.
Pre-Built Testing Actions
You can save time by using pre-configured actions to integrate popular testing frameworks like Jest, Cypress, and Python’s Pytest. Here’s an example:
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Run Jest Tests
uses: actions/jest-runner@v1
with:
config: jest.config.js
- name: Run Cypress Tests
uses: cypress-io/github-action@v5
with:
browser: chrome
record: true
- name: Run Python Tests
uses: actions/setup-python@v4
with:
python-version: '3.11'
- run: |
pip install pytest
pytest tests/
Managing Test Variables
Keep your test credentials and configurations secure. GitHub Actions offers two ways to handle sensitive data:
Variable Type | Use Case | Access Method | Encryption |
---|---|---|---|
Environment Variables | Non-sensitive config | ${{ env.VARIABLE_NAME }} | Plain text |
Secrets | API keys, passwords | ${{ secrets.SECRET_NAME }} | Encrypted |
Here’s how you can set up these variables in your workflow:
jobs:
test:
env:
TEST_ENV: 'staging'
API_URL: 'https://api.staging.example.com'
steps:
- name: Run Integration Tests
env:
API_KEY: ${{ secrets.API_KEY }}
DATABASE_URL: ${{ secrets.DB_CONNECTION }}
run: npm run test:integration
Running Tests on Multiple Systems
Matrix testing allows you to test your application across different environments, such as various Node.js versions and databases. Here’s an example setup:
jobs:
test:
strategy:
matrix:
node-version: [14.x, 16.x, 18.x]
database: [mysql, postgres]
include:
- database: mysql
db_port: 3306
- database: postgres
db_port: 5432
steps:
- name: Setup Node.js ${{ matrix.node-version }}
uses: actions/setup-node@v3
with:
node-version: ${{ matrix.node-version }}
- name: Setup Database
run: |
docker run -d -p ${{ matrix.db_port }}:${{ matrix.db_port }} ${{ matrix.database }}
- name: Run Tests
run: npm test
This configuration ensures your tests are run in parallel across different Node.js versions and database setups, providing thorough coverage for your application.
Making Tests Run Faster
Speed up your tests in GitHub Actions by using parallel execution, caching, and artifact storage.
Running Tests in Parallel
Distribute your tests across multiple runners using a matrix strategy. This approach splits your workload, significantly cutting down execution time:
jobs:
test:
runs-on: ubuntu-latest
strategy:
matrix:
shard: [1, 2, 3, 4]
steps:
- name: Execute Test Shard
run: |
npm run test --shard=${{ matrix.shard }}/4
This method ensures that each shard processes a portion of the tests, maximizing efficiency.
Speed Up Tests with Caching
Eliminate repetitive downloads and installations by caching dependencies and test data. Here’s how you can set it up:
jobs:
test:
steps:
- name: Cache Dependencies
uses: actions/cache@v3
with:
path: |
~/.npm
node_modules
key: ${{ runner.os }}-npm-${{ hashFiles('**/package-lock.json') }}
restore-keys: |
${{ runner.os }}-npm-
- name: Cache Test Results
uses: actions/cache@v3
with:
path: .jest-cache
key: ${{ runner.os }}-jest-${{ hashFiles('**/*.test.js') }}
By caching both dependencies and test results, you can avoid unnecessary steps in future runs.
Saving Test Results
Keep your test results organized for easier debugging and analysis. Here’s how to store and upload them:
jobs:
test:
steps:
- name: Run Tests
run: |
mkdir -p test-results
npm test --json --outputFile=./test-results/junit.xml
- name: Upload Test Results
uses: actions/upload-artifact@v3
with:
name: test-results
path: test-results
retention-days: 14
This setup saves your test outputs and makes them accessible for review, helping you identify and fix issues faster.
Power Features for Testing
Take GitHub Actions to the next level with advanced testing features. These tools go beyond basic workflows, allowing you to fine-tune your testing pipeline for more complex scenarios.
Building Custom Test Actions
Create custom test actions using JavaScript or Docker to automate specialized testing needs. For example, here’s how you can set up cross-browser tests:
name: Browser Compatibility Test
description: 'Runs cross-browser tests using multiple configurations'
inputs:
browsers:
description: 'Browser configurations to test'
required: true
test-suite:
description: 'Path to test suite'
required: true
runs:
using: 'node16'
main: 'index.js'
The corresponding JavaScript implementation:
const core = require('@actions/core');
const exec = require('@actions/exec');
async function run() {
try {
const browsers = core.getInput('browsers').split(',');
const testSuite = core.getInput('test-suite');
for (const browser of browsers) {
await exec.exec(`npm run test:${browser} ${testSuite}`);
}
} catch (error) {
core.setFailed(error.message);
}
}
run();
This setup automates browser-specific tests, ensuring your application behaves consistently across different environments. To go further, leverage matrix configurations for even broader testing coverage.
Matrix Testing Setup
Matrix configurations allow you to run tests across multiple environments efficiently. Here’s an example for running tests across operating systems, Node.js versions, and browsers:
jobs:
test:
strategy:
matrix:
os: [ubuntu-latest, windows-latest, macos-latest]
node: [14, 16, 18]
browser: [chrome, firefox, safari]
exclude:
- os: windows-latest
browser: safari
runs-on: ${{ matrix.os }}
steps:
- uses: actions/checkout@v3
- name: Setup Node.js
uses: actions/setup-node@v3
with:
node-version: ${{ matrix.node }}
- name: Run Tests
run: npm test
This configuration creates 27 different test combinations, enabling thorough validation of your application across diverse environments.
Summary
Key Features and Advantages
GitHub Actions brings testing directly into development workflows, saving time and boosting team productivity by up to 30%. Its seamless integration with GitHub and built-in tools make it a go-to solution for developers.
Here’s how GitHub Actions improves testing:
Feature | Benefit |
---|---|
Native GitHub Integration | Cuts down setup time |
Built-in Platform Tools | Supports multi-environment testing |
Pre-built Actions | Makes tool integration easier |
“GitHub Actions allows us to automate our testing workflows seamlessly, integrating directly with our codebase and improving our deployment speed.” - Jane Doe, Senior Developer at Tech Innovations
These features simplify the creation of reliable and efficient testing pipelines.
How to Get Started
Maximize the potential of GitHub Actions by taking these steps:
- Create a workflow file for your most common tests.
- Explore and add pre-built actions from the GitHub Marketplace.
- Implement caching to speed up build times.
- Use matrix builds to test across multiple environments simultaneously.
With its YAML-based configuration, GitHub Actions makes it easy to define and maintain testing pipelines while ensuring they run efficiently and cover all necessary scenarios.