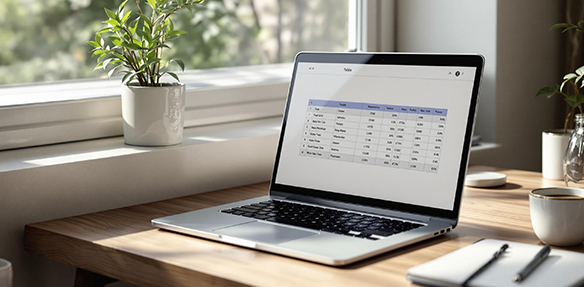
Responsive tables are essential for mobile users, as over 60% of web traffic now comes from mobile devices. Traditional tables often break on small screens, causing frustrating horizontal scrolling and poor readability. This guide shows you how to fix that using CSS Grid and Tailwind CSS.
Key Steps:
- Set Up Tailwind CSS: Install and configure it for your project.
- Build a Semantic Table Structure: Use accessible HTML with Tailwind utilities for responsive padding, spacing, and overflow handling.
- Use CSS Grid for Flexibility: Create layouts that adapt to screen sizes, turning rows into card-like blocks for mobile.
- Mobile-First Design: Stack table data vertically for small screens, then add columns back for larger devices with Tailwind’s breakpoints.
- Improve Usability: Add ARIA labels, keyboard navigation, and lazy loading for better accessibility and performance.
Quick Comparison: CSS Grid vs. Tailwind CSS
Feature | CSS Grid Contribution | Tailwind CSS Advantage |
---|---|---|
Layout Control | Dynamic column adjustments | Pre-built responsive utilities |
Mobile Adaptation | Stacking for small screens | Mobile-first breakpoint system |
Accessibility | Preserves semantic structure | Built-in accessibility features |
Starting Your Project
Kicking off a responsive table project with Tailwind CSS involves setting up your development environment and building a solid HTML foundation. Here’s how to get started.
Setting Up Tailwind CSS
Follow these steps to set up Tailwind CSS:
- Create a new project directory, initialize it with npm, and install Tailwind CSS:
mkdir build-components-using-tailwind
cd build-components-using-tailwind
npm init -y
npm install tailwindcss
- Generate a Tailwind configuration file:
npx tailwindcss init
- Add the following lines to a
tailwind.css
file to include Tailwind’s core styles:
@tailwind base;
@tailwind components;
@tailwind utilities;
Creating the Basic Table Structure
Start by creating a semantic HTML structure for accessibility and easy styling:
<div class="flow-root">
<div class="overflow-x-auto">
<div class="inline-block min-w-full py-2 align-middle sm:px-6 lg:px-8">
<table class="min-w-full divide-y divide-base-300">
<thead>
<tr>
<th scope="col" class="py-3.5 pl-4 pr-3 text-left text-sm font-medium text-base-500 sm:pl-0">Name</th>
<th scope="col" class="px-3 py-3.5 text-left text-sm font-medium text-base-500">Title</th>
<th scope="col" class="px-3 py-3.5 text-left text-sm font-medium text-base-500">Status</th>
</tr>
</thead>
<tbody class="divide-y divide-base-50 bg-white">
<!-- Table rows will go here -->
</tbody>
</table>
</div>
</div>
</div>
This structure includes:
flow-root
: Creates an independent positioning context.overflow-x-auto
: Adds horizontal scrolling for smaller screens.- Responsive padding classes like
sm:px-6
andlg:px-8
to adjust spacing for different screen sizes. - Semantic elements like
<thead>
and<tbody>
to ensure accessibility.
Tailwind CSS provides two key table-specific utilities:
table-auto
: Adjusts column widths dynamically based on content.table-fixed
: Enforces strict column width control.
This setup lays the groundwork for a responsive table. You can further enhance it with CSS Grid and Tailwind’s utility classes to make your tables even more adaptable.
Using CSS Grid for Responsive Tables
CSS Grid, paired with Tailwind CSS, provides an effective way to create tables that look great and work well on any device. Together, these tools make it easy to design layouts that adjust beautifully to different screen sizes.
CSS Grid Basics for Tables
CSS Grid helps you build flexible layouts, whether you’re designing a traditional table structure or a card-style layout for smaller screens. Here’s how you can set it up:
.responsive-table {
display: grid;
grid-template-columns: repeat(auto-fill, minmax(200px, 1fr));
gap: 1rem;
}
The minmax()
function ensures that columns adapt to available space while maintaining a minimum width.
For mobile devices, where space is tight, you can transform table rows into card-like blocks for better readability:
@media (max-width: 640px) {
.table-row {
display: grid;
grid-template-columns: 1fr;
border: 1px solid #e2e8f0;
padding: 1rem;
margin-bottom: 1rem;
}
}
This approach makes data easier to digest on small screens.
Tailwind CSS Grid Utilities
Tailwind CSS speeds up the process with its utility-first approach. Here’s an example of how you can use it:
<div class="grid grid-cols-1 md:grid-cols-3 lg:grid-cols-4 gap-4">
<div class="p-4 bg-white shadow rounded">
<div class="font-bold">Product Name</div>
<div class="text-gray-600">Description</div>
<div class="text-right">Price</div>
</div>
<!-- Add more items as needed -->
</div>
Tailwind’s responsive classes, like md:grid-cols-3
for medium screens and lg:grid-cols-4
for larger screens, make it simple to adjust layouts for different devices.
“CSS Grid is a powerful 2-dimensional layout system, handling both rows and columns, unlike flexbox, which is primarily 1-dimensional.”
Tailwind also offers utilities for spacing, such as gap-x-4
for horizontal spacing and gap-y-2
for vertical spacing. These help keep your table design clean and consistent:
<div class="grid gap-x-4 gap-y-2 md:gap-6">
<!-- Table content -->
</div>
Designing for Mobile and Multiple Devices
Mobile-First Table Design
Creating tables for mobile devices often requires a single-column layout. This approach stacks table data vertically, making it easier to read on smaller screens. By turning rows into card-like components, each piece of data gets its own space, avoiding the hassle of horizontal scrolling:
<div class="grid grid-cols-1 gap-4 p-4">
<div class="bg-white rounded-lg shadow p-4">
<div class="font-bold text-gray-700">Product Name</div>
<div class="text-gray-600">Description</div>
<div class="mt-2 text-right">Price</div>
</div>
</div>
Pair semantic HTML with Tailwind’s utility classes to ensure your design is both clean and accessible:
<div role="table" aria-label="Products" class="grid grid-cols-1 gap-4">
<div role="row" class="bg-white p-4 rounded shadow">
<div role="cell" class="font-medium text-gray-900">Cell Content</div>
</div>
</div>
Adding Breakpoints for Larger Screens
Tailwind’s breakpoint utilities make it easy to adapt your tables for larger screens. You can progressively add columns, starting with one on mobile and scaling up to four on desktops:
<div class="grid
grid-cols-1
sm:grid-cols-2
md:grid-cols-3
lg:grid-cols-4
gap-4 p-4"
>
<!-- Table content -->
</div>
For more complex tables, consider using conditional rendering. This allows you to show detailed views on larger screens while keeping things simple on mobile:
<div class="hidden md:block">
<!-- Detailed view for larger screens -->
</div>
<div class="md:hidden">
<!-- Simplified view for mobile -->
</div>
To maintain consistent spacing, use Tailwind’s gap utilities like gap-x-4
and gap-y-2
. These help ensure that your layout remains visually balanced as you add more columns:
<div class="grid
grid-cols-1
md:grid-cols-3
gap-x-4
gap-y-2
md:gap-6"
>
<!-- Table content -->
</div>
With these responsive techniques in place, you can focus on improving usability and fine-tuning performance to deliver a smooth experience across all devices.
Improving Usability and Performance
Making Tables More Accessible
Did you know that over 71% of users with disabilities depend on assistive technologies? [1] Ensuring tables are accessible is a key step in creating responsive designs that work for everyone. Here are some practical ways to make your tables more inclusive:
- ARIA Labels: Add
aria-label
attributes to describe the table’s purpose. For example:
<table role="table" aria-label="Product Inventory">
<tr role="row">
<th role="columnheader" scope="col">Product</th>
</tr>
</table>
- Keyboard Navigation: Use proper
tabindex
values to ensure users can navigate logically through the table. - Data Labels: Use
data-label
attributes to keep context clear, especially on mobile:
<td role="cell" data-label="Product">Item Name</td>
These adjustments are especially helpful for mobile users, where responsive layouts can sometimes make important details harder to follow. By addressing accessibility, you’re improving usability for everyone.
Boosting Table Performance
Large tables can slow down page load times, particularly on mobile devices. And here’s why that matters: Amazon found that even a 1-second delay in load time can cut conversions by 7%. To avoid this, follow these steps to improve table performance:
- Lazy Loading: Use the Intersection Observer API to load table rows only when they’re visible. This reduces initial load time and conserves memory.
- Smart Styling: Take advantage of utility classes like those in Tailwind CSS to manage styling efficiently:
<div class="overflow-auto">
<table class="min-w-full divide-y divide-gray-200">
<tbody class="divide-y divide-gray-200">
<!-- Dynamically loaded content -->
</tbody>
</table>
</div>
This approach not only ensures smooth scrolling but also optimizes memory use, which is critical for devices with limited resources [2]. By focusing on both usability and performance, your tables will be ready to meet the expectations of today’s web users.
Testing and Debugging Tables
Testing tables on various devices ensures they work well and align with design goals. Here’s how to test and troubleshoot effectively.
Testing and Debugging Responsive Tables
Chrome DevTools is a handy tool for examining responsive tables. Its Layout panel lets you visualize grid structures by enabling grid overlays on elements using display: grid
. For more thorough testing, platforms like Testsigma allow cross-device testing on over 2000 iOS and Android devices, making it easier to handle complex layouts.
Here are some quick fixes for common mobile table issues:
<!-- Horizontal Overflow -->
<div class="overflow-auto">
<table class="min-w-full">
<!-- Table content -->
</table>
</div>
<!-- Grid Alignment -->
<div class="grid grid-cols-1 sm:grid-cols-2 md:grid-cols-3 gap-4">
<!-- Table content -->
</div>
When testing responsive tables, keep these tips in mind:
- Use real content instead of placeholder text.
- Confirm that keyboard navigation is smooth and functional.
- Test table behavior at different screen widths.
- Check compatibility across multiple browsers.
Additionally, tools like NVDA or VoiceOver can help ensure table content remains accessible and clear when adjusted for mobile layouts. These strategies will help you create tables that work well on any device.
Conclusion: Key Points and Next Steps
Best Practices Summary
Building responsive tables starts with a mobile-first approach, using CSS Grid and Tailwind utilities to create layouts that adapt well to different devices. This method ensures your tables stay easy to use and accessible, without sacrificing performance or usability.
With these basics in place, it’s time to look at tools that can make your responsive table designs even better.
Additional Resources and Learning
Using the right tools can simplify your workflow and solve common issues with responsive tables. For instance, Chrome DevTools helps you visualize grids and debug layouts, while the Tailwind CSS documentation offers practical guidance for responsive design. To test your designs across multiple devices, Hoverify can save time and effort.
Here’s a quick overview of these tools:
Tool | Purpose |
---|---|
Chrome DevTools | Visualize grids and debug layouts |
Tailwind CSS Docs | Explore responsive design techniques |
Hoverify | Preview designs on multiple devices |
These tools are especially helpful for solving problems like grid alignment, breakpoint testing, and ensuring accessibility on different screens.
Keep in mind, creating responsive tables is an ongoing process. Regular testing, gathering user feedback, and tweaking based on performance data will help you deliver tables that look great and work well on any device.
FAQs
How to make a table responsive using Tailwind?
Creating responsive tables in Tailwind CSS involves using utility classes to adapt the table layout for different screen sizes. Here’s an example:
<div class="overflow-x-auto">
<table class="min-w-full border-collapse block md:table">
<thead class="block md:table-header-group">
<tr class="border border-gray-200 md:border-none block md:table-row">
<th class="p-2 text-left font-medium md:border md:border-gray-200 block md:table-cell">Name</th>
<th class="p-2 text-left font-medium md:border md:border-gray-200 block md:table-cell">Email</th>
<th class="p-2 text-left font-medium md:border md:border-gray-200 block md:table-cell">Role</th>
</tr>
</thead>
<tbody class="block md:table-row-group">
<!-- Table content -->
</tbody>
</table>
</div>
To ensure the table works well across devices, follow these steps:
- Use utilities like
block md:table
to toggle between stacked and table layouts based on screen size. - Add
border-separate
andborder-spacing-y-2
for consistent row spacing.
“We need to add some spacing between each of the rows which we can do using Tailwind’s Border spacing for tables. Let’s also add the default text size of small and wrap the table in a div so we can center it using flex.” - Daniel Salvado, a software developer and author at Evodeck Software, highlights the importance of spacing and alignment in responsive table design [2].
Additionally, use whitespace-nowrap
to avoid text wrapping in cells, ensuring the table remains clean and easy to read on smaller screens. This method keeps your tables functional and visually appealing across all devices.