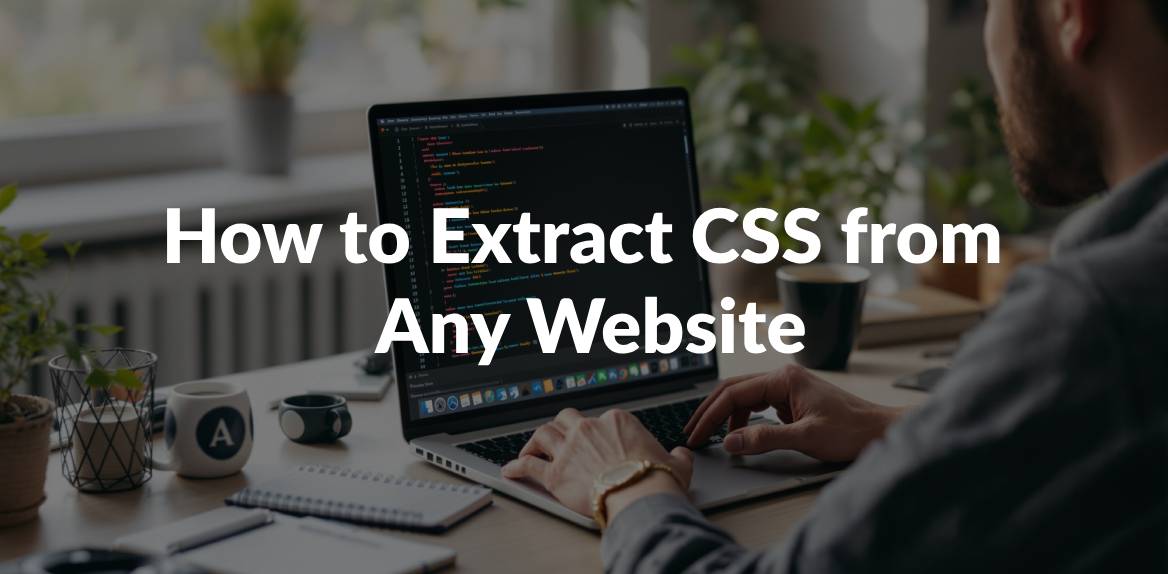
Want to extract CSS from any website quickly and easily? Here’s how:
- Use Browser Developer Tools: Access tools like Chrome’s “Elements” panel to inspect and copy CSS styles.
- Download Full Stylesheets: Use the “Network” or “Sources” panel to locate and save CSS files.
- Try Browser Extensions: Tools like Hoverify let you hover over elements, view, and export CSS instantly.
- Leverage Command Line Tools: Automate CSS extraction for multiple pages using Node.js and Puppeteer.
Important: Always respect copyright laws and use extracted CSS for learning or debugging unless you have permission for commercial use.
This guide covers step-by-step methods for CSS extraction, tools, and best practices for managing your styles efficiently.
Browser Developer Tools Method
This approach uses browser developer tools to efficiently extract and analyze CSS styles from any website. These tools provide a detailed view of styles and allow precise control over what you want to inspect.
Opening Developer Tools
Here’s how to access developer tools in different browsers:
- Chrome: Press F12 or Ctrl+Shift+I (Cmd+Option+I on Mac)
- Firefox: Press F12 or right-click on an element and choose “Inspect Element”
- Safari: Enable developer tools in Preferences > Advanced, then press Cmd+Option+I
Once opened, go to the “Elements” panel in Chrome or Safari, or the “Inspector” panel in Firefox, to view the website’s HTML structure and styles.
Finding and Copying CSS
Follow these steps to extract specific CSS styles:
1. Select the Element
Click the element picker icon (a small cursor icon) in the developer tools, then click on the element you want to inspect. This highlights its associated CSS.
2. View Computed Styles
Go to the “Computed” tab to see the final CSS values applied to the element, including inherited styles.
3. Copy CSS Rules
Right-click on a CSS rule in the “Styles” or “Computed” panel to copy either a specific declaration or the entire rule.
If you need more, you can extend this process to download entire CSS files.
Getting Complete Stylesheets
To download full CSS files, use the Network or Sources panel:
- Network Panel: Filter requests by “CSS”, then right-click the desired file to copy its link or save it directly.
- Sources Panel: Locate .css files and download them from here.
Tip: If the CSS file is minified, use the “Pretty print” feature (often displayed as a {}
icon) to reformat the code for easier reading.
Keep in mind that large websites often use multiple stylesheets or load CSS dynamically. Use the Network panel to monitor all CSS files loaded during page interactions to ensure you capture everything.
Browser Extensions for CSS Extraction
Browser extensions can simplify CSS extraction by automating tasks and enabling real-time editing, saving developers time and effort.
Hoverify CSS Tools
Hoverify is a browser extension designed to simplify CSS inspection and extraction. Its Inspector tool lets you view CSS styles by simply hovering over elements, eliminating the need for manual inspection.
Here’s what Hoverify’s CSS tools offer:
- View styles instantly by hovering over elements
- Edit CSS in real time
- Extract styles at the component level
- Identify color palettes
- Search elements by tag, ID, or class
How to Use Hoverify for CSS Extraction
Follow these steps to extract CSS using Hoverify:
-
Activate Inspector Mode
Click the Hoverify icon in your browser to enable Inspector Mode. As you hover over elements, they’ll be highlighted for easy inspection. -
Extract Styles
Hover over any element to see its CSS properties. From there, you can:- Copy specific properties
- Export components with their CSS
- Extract entire style blocks
- Save styles directly to CodePen
-
Edit and Test
Hoverify allows you to tweak CSS in real time, so you can make changes and test them immediately.
Hoverify doesn’t just simplify CSS extraction - it also includes additional tools to boost your overall productivity.
Additional Developer Features
Hoverify goes beyond CSS with tools for asset management and debugging:
Asset Management
- Extract images, SVGs, and other assets
- Collect and identify page colors
- Export components along with assets
Development Tools
- Test responsive designs across different devices
- Debug CSS issues as they occur
- Analyze media queries and animations
Hoverify is trusted by over 22,000 developers [2] and works with popular browsers like Chrome, Firefox, and Chromium-based browsers such as Brave and Edge [1]. To ensure smooth performance, keep the extension updated. If you run into problems, uninstalling and reinstalling the extension often resolves most issues while giving you access to the latest features and fixes [2].
Command Line CSS Extraction
Command line tools simplify the process of extracting CSS from websites, making them especially useful for handling multiple pages or complex web apps.
Common CLI Tools
These tools often rely on headless browsers and web scraping libraries. Many are built on Node.js, providing programmatic control over the extraction process.
Here’s an example script using Node.js and Puppeteer to extract CSS:
const puppeteer = require('puppeteer');
async function extractCSS(url) {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto(url);
const styles = await page.evaluate(() => {
const styleSheets = Array.from(document.styleSheets);
return styleSheets.map(sheet => {
try {
return Array.from(sheet.cssRules)
.map(rule => rule.cssText)
.join('\n');
} catch (e) {
return '';
}
}).join('\n');
});
await browser.close();
return styles;
}
When working with command line tools, keep these in mind:
- Set timeouts for page loading
- Handle authentication if required
- Configure proxy settings when needed
- Enable JavaScript execution for dynamic content
- Adjust viewport settings for accurate rendering
Batch CSS Extraction
For large-scale projects, batch scripts can automate CSS extraction across multiple URLs. Here’s a sample script for batch processing:
const fs = require('fs');
const path = require('path');
async function batchExtract(urlList) {
const results = {};
for (const url of urlList) {
const css = await extractCSS(url);
const filename = `${path.parse(url).name}.css`;
fs.writeFileSync(filename, css);
results[url] = filename;
}
return results;
}
For effective batch extraction, follow these tips:
- Organize URLs: Group pages by section or functionality to streamline the process and maintain consistent file naming.
- Handle Errors: Add retries for failed attempts and log errors without halting the entire operation.
- Boost Performance: Use concurrent processing, set rate limits, and cache shared resources to save time.
- Post-Process CSS: Remove duplicates, merge similar selectors, minify files, and create source maps for easier use.
Integrating CSS extraction into build processes or CI pipelines can automate style management during development. Up next, explore ways to efficiently manage your extracted CSS.
CSS Management Guidelines
Effectively managing CSS is key to ensuring smooth integration and optimal performance in your projects.
How to Organize CSS Files
Keep your CSS structured in a clear and logical way. Here’s a common hierarchy to follow:
- Base: Includes foundational files like
reset.css
,typography.css
, andvariables.css
. - Components: Contains reusable elements such as
buttons.css
,forms.css
, andnavigation.css
. - Layout: Focuses on structural files like
grid.css
,header.css
, andfooter.css
.
To keep things tidy:
- Group selectors that are related.
- Stick to consistent naming conventions like BEM or SMACSS.
- Add comments to explain complex selectors.
- Arrange vendor prefixes in an orderly manner.
A well-organized CSS structure not only simplifies collaboration but also improves efficiency.
Tips for Boosting CSS Performance
- Minify your CSS: Tools like
cssnano
orclean-css
can help reduce file size. - Combine files: Consolidate multiple CSS files to cut down on HTTP requests.
- Regularly audit your CSS: Identify and remove unused styles to keep it lean.
Tackling Common CSS Challenges
CSS issues can crop up even with good organization. Here’s how to deal with typical problems:
- Style Conflicts: Use precise selectors and a reset stylesheet to avoid unexpected overlaps.
- Browser Compatibility: Add vendor prefixes and test your styles across different browsers for consistency.
- Code Maintenance: Periodically review your CSS to ensure it remains clean and efficient.
Summary
Extracting CSS has become simpler and more efficient with tools like browser developer tools, extensions such as Hoverify, and command-line utilities. These solutions cater to a range of needs, from basic CSS extraction to streamlining workflows for web developers.
Web developer Madhu Menon highlights Hoverify’s ability to inspect designs and extract assets with ease.
To maintain a smooth CSS workflow, consider these practices:
- Stay organized: Group related styles and use a clear file structure.
- Boost performance: Minify CSS files and combine them to cut down on HTTP requests.
- Test extensively: Verify that extracted styles function properly across various browsers and devices.
- Keep track of changes: Use comments to clarify complex selectors and document modifications.
With tools like Hoverify and browser developer utilities, CSS extraction not only saves time but also supports clean, efficient code - whether you’re managing small projects or large-scale websites.