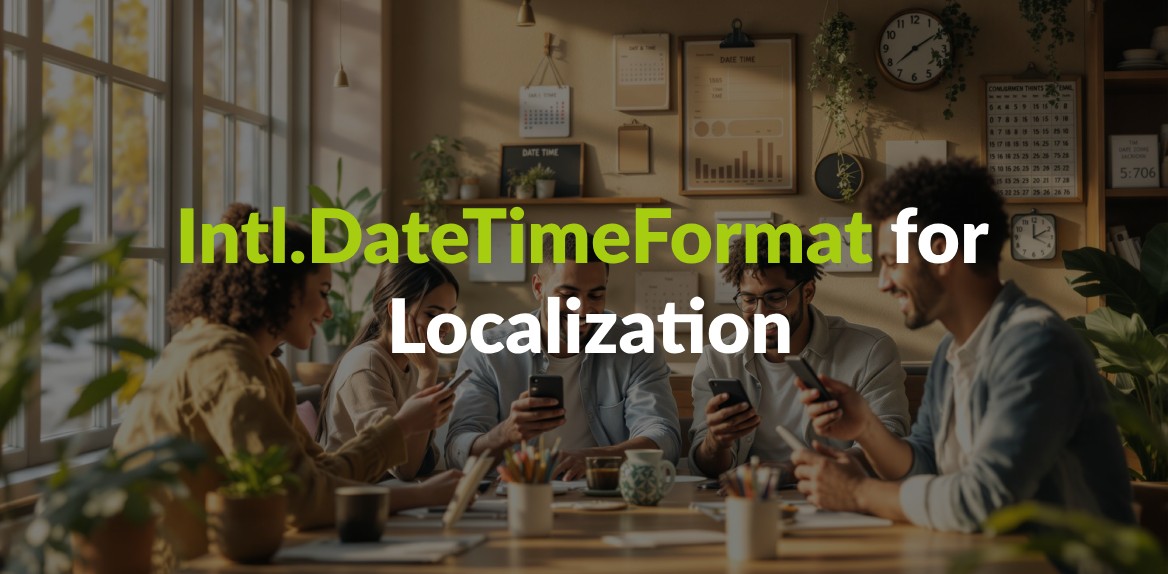
Want to display dates and times in a way that makes sense for users worldwide? JavaScript’s Intl.DateTimeFormat
API makes it easy. Here’s why it matters and how to use it:
- What it does: Automatically formats dates and times based on user language and region.
- Why it’s useful:
- Displays dates in familiar formats (e.g., “03/28/2025” in the US, “28/03/2025” in Europe).
- Saves time by eliminating the need for custom formatting code.
- Handles regional quirks like date order, time zones, and daylight saving time.
- How to use it: Create a formatter with a locale (e.g.,
en-US
) and options likedateStyle
andtimeStyle
.
Example:
const formatter = new Intl.DateTimeFormat('en-US', { dateStyle: 'full' });
console.log(formatter.format(new Date())); // Output: Friday, March 28, 2025
Key Features:
- Supports over 150 locales.
- Built-in time zone handling with IANA identifiers.
- Flexible customization for date and time components.
Whether you’re building a global app or handling time zones, Intl.DateTimeFormat
ensures accurate, user-friendly date localization. Let’s explore how to implement it effectively.
Getting Started with Intl.DateTimeFormat
Setting Up DateTimeFormat
Creating an Intl.DateTimeFormat
instance is straightforward:
const formatter = new Intl.DateTimeFormat('en-US');
const today = new Date();
console.log(formatter.format(today));
// Output: 3/28/2025
You can also customize the output with additional options:
const formatter = new Intl.DateTimeFormat('en-US', {
dateStyle: 'full',
timeStyle: 'long'
});
console.log(formatter.format(today));
// Output: Friday, March 28, 2025 at 2:30:45 PM EDT
Using the format() Method
The format()
method transforms Date
objects into localized strings:
const formatter = new Intl.DateTimeFormat('en-US', {
year: 'numeric',
month: 'long',
day: 'numeric'
});
// Current date
console.log(formatter.format(new Date()));
// Output: March 28, 2025
// Specific date
const christmas = new Date(2025, 11, 25);
console.log(formatter.format(christmas));
// Output: December 25, 2025
You can fine-tune the output by specifying locale settings to match regional preferences.
Setting Language and Region
Here are some common locale options:
- ‘en-US’: American English (e.g., 3/28/2025)
- ‘en’: Generic English, follows system defaults
- ‘en-US-u-ca-gregory’: US English using the Gregorian calendar
Here’s an example of customizing the output with locale settings:
const formatter = new Intl.DateTimeFormat('en-US', {
weekday: 'long',
year: 'numeric',
month: 'short',
day: 'numeric',
hour: 'numeric',
minute: 'numeric',
timeZone: 'America/New_York'
});
console.log(formatter.format(new Date()));
// Output: Friday, Mar 28, 2025, 2:30 PM
For applications supporting multiple regions, you can create separate formatters for each locale:
const formatters = {
us: new Intl.DateTimeFormat('en-US'),
uk: new Intl.DateTimeFormat('en-GB'),
jp: new Intl.DateTimeFormat('ja-JP')
};
const date = new Date();
console.log(formatters.us.format(date)); // 3/28/2025
console.log(formatters.uk.format(date)); // 28/03/2025
console.log(formatters.jp.format(date)); // 2025/3/28
Modern browsers handle region-specific rules for date components, separators, and time formats automatically. By following these steps, you can reliably format dates for any locale, ensuring your applications meet global requirements.
Format Customization Options
Basic Formatting Settings
The Intl.DateTimeFormat
constructor lets you adjust the format with two main options: dateStyle
and timeStyle
. These presets follow local conventions:
const formatter = new Intl.DateTimeFormat('en-US', {
dateStyle: 'full',
timeStyle: 'medium'
});
console.log(formatter.format(new Date()));
// Output: Friday, March 28, 2025 at 2:30:45 PM
Here are the available values for these options:
- full: Provides the most detailed output.
- long: Includes more details than necessary for everyday use.
- medium: Offers a standard format with key elements.
- short: A compact version, perfect for tight spaces.
For more specific needs, you can customize individual date and time components.
Date and Time Components
If you need precise control, you can define each component individually:
const formatter = new Intl.DateTimeFormat('en-US', {
year: 'numeric',
month: 'long',
day: '2-digit',
hour: 'numeric',
minute: '2-digit',
second: '2-digit',
hour12: true,
fractionalSecondDigits: 3
});
Here’s how the component options work:
- numeric: Displays numbers like 3, 28, 2025.
- 2-digit: Uses two digits, such as 03, 28, 25.
- long: Outputs full names, like March or Friday.
- short: Abbreviates names to Mar or Fri.
- narrow: Uses minimal letters, like M or F.
Format Examples
Below are some examples of how you can use these settings for different scenarios:
// Short date for forms
const shortDate = new Intl.DateTimeFormat('en-US', {
month: 'numeric',
day: 'numeric',
year: '2-digit'
});
// Output: 3/28/25
// Full timestamp for logging
const timestamp = new Intl.DateTimeFormat('en-US', {
year: 'numeric',
month: '2-digit',
day: '2-digit',
hour: '2-digit',
minute: '2-digit',
second: '2-digit',
hour12: false,
timeZoneName: 'short'
});
// Output: 03/28/2025, 14:30:45 EDT
// Calendar display
const calendar = new Intl.DateTimeFormat('en-US', {
weekday: 'short',
month: 'short',
day: 'numeric'
});
// Output: Fri, Mar 28
For official documents like contracts or reports:
const formal = new Intl.DateTimeFormat('en-US', {
year: 'numeric',
month: 'long',
day: 'numeric',
weekday: 'long'
});
// Output: Friday, March 28, 2025
This customization allows you to create formats for a variety of purposes, setting the foundation for handling time zones in the next section.
Time Zone Management
Managing time zones accurately is crucial for showing localized dates correctly in global applications.
Setting Time Zones
The timeZone
option, paired with IANA identifiers, makes it easy to format dates for specific regions:
const nyFormatter = new Intl.DateTimeFormat('en-US', {
dateStyle: 'full',
timeStyle: 'long',
timeZone: 'America/New_York'
});
const date = new Date();
console.log(nyFormatter.format(date));
// Output: Friday, March 28, 2025 at 2:30:45 PM EDT
You can also control how time zone names are displayed using the timeZoneName
option:
const formatter = new Intl.DateTimeFormat('en-US', {
dateStyle: 'full',
timeZoneName: 'longOffset'
});
// Output: Friday, March 28, 2025, GMT-04:00
const shortFormatter = new Intl.DateTimeFormat('en-US', {
dateStyle: 'full',
timeZoneName: 'short'
});
// Output: Friday, March 28, 2025, EDT
UTC vs Local Time
To see the difference between UTC and local time, compare their formatting. Use timeZone: 'UTC'
for consistent UTC-based output:
const utcFormatter = new Intl.DateTimeFormat('en-US', {
dateStyle: 'full',
timeStyle: 'long',
timeZone: 'UTC'
});
const localFormatter = new Intl.DateTimeFormat('en-US', {
dateStyle: 'full',
timeStyle: 'long'
});
const date = new Date();
console.log(utcFormatter.format(date));
// Output: Friday, March 28, 2025 at 6:30:45 PM UTC
console.log(localFormatter.format(date));
// Output: Friday, March 28, 2025 at 2:30:45 PM EDT
Common Time Zone Issues
Real-world applications often face challenges when working with time zones. Here’s how to address some of the most common problems:
Issue | Solution |
---|---|
Daylight Saving Time (DST) transitions | Use the timeZone option with IANA identifiers to handle DST automatically |
Invalid times during DST changes | Add error handling for dates that don’t exist (e.g., during spring forward) |
Ambiguous times during fall back | Use exact UTC timestamps to avoid confusion |
Browser time zone detection | Depend on system settings instead of manual detection |
For example, handling DST transitions can be tricky:
const formatter = new Intl.DateTimeFormat('en-US', {
dateStyle: 'full',
timeStyle: 'long',
timeZone: 'America/New_York'
});
// Handle spring forward (2:00 AM becomes 3:00 AM)
const springForward = new Date('2025-03-09T02:30:00');
try {
console.log(formatter.format(springForward));
} catch (error) {
console.log('Invalid time during DST transition');
}
// Handle fall back (1:00 AM occurs twice)
const fallBack = new Date('2025-11-02T01:30:00Z');
console.log(formatter.format(fallBack));
To avoid confusion, store all timestamps in UTC and convert them to local time only when displaying them. This approach ensures consistency and prevents ambiguity.
Date Localization Guidelines
Selecting Target Regions
Use navigator.language
to detect the user’s locale and set a default fallback:
const userLocale = navigator.language || 'en-US'; // Fallback to 'en-US'
const formatter = new Intl.DateTimeFormat(userLocale, {
dateStyle: 'full',
timeStyle: 'long'
});
Define your primary locales based on the regions you are targeting:
Region | Locale Code | Date Format Example |
---|---|---|
United States | en-US | March 28, 2025 |
United Kingdom | en-GB | 28 March 2025 |
Germany | de-DE | 28. März 2025 |
Japan | ja-JP | 2025年3月28日 |
This list helps you manage unsupported regions by providing fallback options.
Handling Unsupported Regions
For unsupported locales, implement a fallback mechanism:
function createSafeFormatter(locale, options) {
try {
return new Intl.DateTimeFormat(locale, options);
} catch (error) {
// Try language-only fallback
const languageOnly = locale.split('-')[0];
try {
return new Intl.DateTimeFormat(languageOnly, options);
} catch (error) {
// Default to 'en-US'
return new Intl.DateTimeFormat('en-US', options);
}
}
}
Fallback strategy:
- Use the exact locale requested.
- Fallback to the base language (e.g., ‘en’ for ‘en-GB’).
- Match the closest supported locale.
- Default to ‘en-US’ as a last resort.
Testing Regional Formats
To ensure accurate formatting, test across various locales:
const testDate = new Date('2025-03-28T14:30:45');
const testLocales = ['en-US', 'en-GB', 'de-DE', 'ja-JP'];
const formatOptions = {
dateStyle: 'full',
timeStyle: 'long'
};
testLocales.forEach(locale => {
const formatter = createSafeFormatter(locale, formatOptions);
console.log(`${locale}: ${formatter.format(testDate)}`);
});
Your tests should cover:
- Date order (e.g., month/day/year vs. day/month/year).
- Time formats (12-hour vs. 24-hour clocks).
- Number separators for decimals and thousands.
- Calendar variations (e.g., Gregorian, Buddhist, Japanese Imperial).
- Special cases like leap years and daylight saving time (DST).
Edge cases to validate include:
const edgeCases = [
new Date(0), // Unix epoch
new Date('2024-02-29'), // Leap year
new Date('2025-12-31T23:59:59'), // Year boundary
new Date('2025-03-09T02:30:00') // DST transition
];
Thorough testing ensures your application displays user-friendly and accurate date formats across all regions.
Conclusion
Benefits of Using DateTimeFormat
Intl.DateTimeFormat simplifies localization by managing formatting rules, time zones, and regional styles automatically. Some of its standout features include support for over 150 locales, automatic handling of complex formatting rules, and consistent behavior across modern browsers. By using this API, you can avoid manual string manipulation and regional inconsistencies, ensuring a standardized and reliable approach to date formatting.
Feature | Description |
---|---|
Locale Detection | Automatically applies the user’s regional preferences |
Format Flexibility | Handles various date/time components and styles |
Time Zone Handling | Supports all IANA time zones out of the box |
Performance | Offers optimized native performance compared to custom-built solutions |
Maintenance | Simplifies code and reduces technical debt |
These features make Intl.DateTimeFormat a practical choice for developers looking to handle date formatting efficiently.
Tips for Implementation
Here are some tips to make the most of Intl.DateTimeFormat:
-
Reuse Formatter Instances: To boost performance, create formatter instances once and reuse them. Here’s an example:
const formatters = new Map(); function getFormatter(locale, options) { const key = `${locale}-${JSON.stringify(options)}`; if (!formatters.has(key)) { formatters.set(key, new Intl.DateTimeFormat(locale, options)); } return formatters.get(key); }
-
Test for Edge Cases: Be sure to check scenarios like daylight saving time transitions, leap years, and date boundaries.
-
Use dateStyle and timeStyle Options: Opt for these built-in options instead of configuring individual components. They simplify setup while ensuring accuracy.