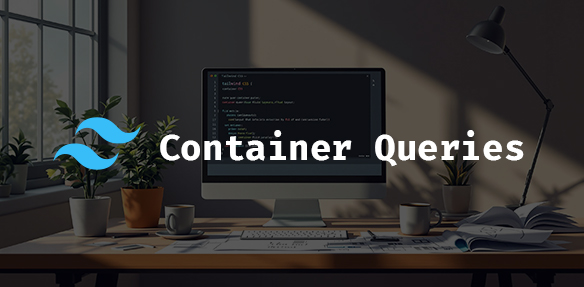
Container queries are changing how we build responsive designs. Unlike media queries, which rely on the entire screen size, container queries let components adapt to their parent container’s size. This makes designs more flexible and easier to maintain, especially when paired with Tailwind CSS.
Key Points:
- What are container queries? They allow components to respond to their container’s size, not the viewport.
- Why switch from media queries? Media queries are global, harder to maintain, and don’t adapt to component contexts.
- How to use in Tailwind? Install the
@tailwindcss/container-queries
plugin, define custom breakpoints, and use classes like@container
and@sm:flex
for responsive designs. - Benefits: Smarter, reusable components and cleaner codebases.
Quick Comparison: Media Queries vs. Container Queries
Feature | Media Queries | Container Queries |
---|---|---|
Scope | Viewport-wide | Parent container-specific |
Reusability | Limited across contexts | Highly reusable |
Context | Blind to parent container size | Fully responsive to container size |
Use Case | Page-wide layout adjustments | Component-level responsiveness |
Container queries fit perfectly with Tailwind’s utility-first approach, enabling fluid layouts with minimal effort. Want to make your designs smarter and more efficient? This guide shows you how.
Setting Up Container Queries in Tailwind
Let’s dive into how to set up container queries in Tailwind’s utility-first framework.
Installing the @tailwindcss/container-queries Plugin
Start by adding the plugin using your preferred package manager:
npm install @tailwindcss/container-queries # or yarn add
Configuring Tailwind for Container Queries
Once installed, you need to configure the plugin to define custom breakpoints that suit your components:
/** @type {import('tailwindcss').Config} */
module.exports = {
plugins: [require('@tailwindcss/container-queries')],
theme: {
containerQueries: {
'custom-sm': '300px',
'custom-md': '500px'
}
}
}
Using Container Queries in Tailwind
To apply container queries, designate an element as a container with the @container
class. Then, use container-specific variants to style elements responsively based on the container’s dimensions:
<div class="@container">
<div class="block @lg:flex gap-4">
<img class="w-full @lg:w-1/2" src="image.jpg" alt="Product">
<div class="@lg:w-1/2">
<h2 class="text-xl @lg:text-2xl">Product Title</h2>
<p class="@lg:text-lg">Description</p>
</div>
</div>
</div>
For more intricate layouts requiring unique breakpoints:
<div class="@container/sidebar">
<nav class="@lg/sidebar:flex-col">
<!-- Sidebar navigation -->
</nav>
</div>
Creating Fluid Layouts with Container Queries
Container queries in Tailwind CSS let you design responsive components that adapt based on their container’s size. Here’s how to put them into action:
Example: Responsive Product Card
Here’s a product card that adjusts its layout depending on the width of its container:
<div class="@container bg-white rounded-lg shadow-md">
<div class="@sm:flex @lg:flex-col p-4">
<img class="@sm:w-1/3 @lg:w-full rounded-lg" src="product-image.jpg" alt="Product">
<div class="@sm:w-2/3 @lg:w-full @sm:pl-4 @lg:pl-0 @lg:pt-4">
<h2 class="@sm:text-lg @lg:text-xl font-bold">Product Title</h2>
<p class="@sm:text-sm @lg:text-base mt-2 text-gray-600">Product description</p>
<div class="@sm:flex @lg:block items-center mt-4">
<span class="@sm:text-lg @lg:text-xl font-bold text-blue-600">$99.99</span>
<button class="@sm:ml-4 @lg:ml-0 @lg:mt-2 bg-blue-500 text-white px-4 py-2 rounded">
Add to Cart
</button>
</div>
</div>
</div>
</div>
By leveraging container queries, the card’s layout can shift seamlessly between different screen sizes without relying solely on global breakpoints.
Using Tailwind Utilities for Container Queries
Tailwind’s utility-first design works perfectly with container queries. You can apply responsive styles directly to elements within a container:
<div class="@container">
<div class="bg-gray-100 p-4 @md:p-6 @lg:p-8 rounded-lg">
<div class="@sm:flex @lg:grid @lg:grid-cols-3 gap-4">
<div class="@sm:w-1/2 @lg:col-span-2">
<h2 class="text-lg @md:text-xl @lg:text-2xl font-bold">
Featured Content
</h2>
<p class="text-sm @md:text-base @lg:text-lg mt-2">
Dynamic content area
</p>
</div>
<aside class="@sm:w-1/2 @lg:col-span-1 mt-4 @sm:mt-0">
<div class="bg-white p-4 rounded">
<h3 class="@md:text-lg font-semibold">Related Items</h3>
</div>
</aside>
</div>
</div>
</div>
This example shows how you can mix and match Tailwind’s utilities with container queries to create layouts that adapt smoothly.
Nested Containers for Complex Architectures
For projects with multi-level components, you can nest containers to handle more intricate layouts:
<div class="@container/main">
<div class="@container/sidebar flex @lg/main:flex-row flex-col">
<aside class="@lg/main:w-1/4 w-full">
<nav class="@md/sidebar:flex @lg/sidebar:block space-y-2">
<a href="#" class="block @md/sidebar:inline-block px-4 py-2 hover:bg-gray-100">
Dashboard
</a>
<a href="#" class="block @md/sidebar:inline-block px-4 py-2 hover:bg-gray-100">
Analytics
</a>
</nav>
</aside>
<main class="@lg/main:w-3/4 w-full @lg/main:pl-6">
<div class="@container/content">
<div class="grid @md/content:grid-cols-2 @lg/content:grid-cols-3 gap-4">
<!-- Content cards -->
</div>
</div>
</main>
</div>
</div>
This setup allows for precise control over how each section responds to its container’s size, making it easier to manage complex layouts while keeping the code organized and readable.
Comparing Container Queries and Media Queries
Now that we’ve explored how container queries work, let’s break down how they compare to traditional media queries.
Container Queries vs Media Queries: A Comparison
Feature | Container Queries | Media Queries |
---|---|---|
Scope | Targets individual components | Applies changes across the viewport |
Context | Adjusts based on parent container size | Responds to device screen width |
Reusability | Highly reusable across contexts | Limited to fixed viewport breakpoints |
Browser Support | Supported in modern browsers | Works across all browsers |
Performance | Slight overhead | Minimal impact |
When to Use Container Queries
Container queries shine in component-driven designs where elements need to respond to their immediate surroundings. They are especially useful for:
- Reusable components: Adapting to different contexts without extra code.
- Dynamic layouts: Adjusting seamlessly based on available space.
For instance, in the product card example, container queries allowed the component to adapt its layout based on its container size, independent of the overall viewport.
Practical Considerations
While container queries are a game-changer for component-level responsiveness, keep these points in mind:
- Browser compatibility: Ensure fallback solutions for older browsers that don’t support container queries.
- Media queries are still essential: Use them for broader layout needs, such as:
- Structuring the page
- Adjusting typography
- Styling for print
- Detecting device capabilities
By combining media queries for global layouts with container queries for individual components, you can create more flexible designs in frameworks like Tailwind.
For testing and debugging these interactions, tools like Hoverify (discussed next) can simplify the process.
Tools for Working with Container Queries
Tailwind’s container query setup works seamlessly with modern debugging tools. A great example is Hoverify (tryhoverify.com), which simplifies container query debugging with features like:
- Real-time Container Visualization: Resize containers dynamically and see layout changes instantly.
- Visual Overlay System: Highlight container boundaries and breakpoints, making it easier to understand nested containers.
- Container-specific Style Inspector: Quickly inspect and tweak container-based styles using a dedicated interface.
- Code Generation: Automatically create container query snippets to speed up your workflow.
These tools align perfectly with Tailwind’s utility-first approach by offering clear visual feedback during development.
Browser DevTools also come with built-in support for container queries. Chrome’s Elements panel includes features such as:
- Monitoring container dimensions in real time.
- Tracking active and inactive query states.
- Highlighting containment contexts directly.
- Testing container sizes interactively.
For teams, browser extensions like Responsive Viewer can enhance Hoverify by enabling simultaneous testing across various container sizes.
Chrome’s Performance panel is another handy tool, helping you pinpoint rendering or layout issues - especially useful for complex nested containers or dynamic layouts.
Finally, cross-browser tools like Firefox’s Inspector offer similar features for container queries, and the Container Query Playground provides a great space to experiment and refine your designs.
Conclusion: The Role of Container Queries in Responsive Design
Container queries have brought a new level of flexibility to responsive design in Tailwind CSS, enabling components to adapt based on their container rather than the viewport. This shift has shown practical benefits in real-world use. For instance, Netlify’s documentation team simplified their CSS while boosting component reuse by integrating container queries.
“Container queries are a game-changer for responsive design, allowing us to create truly reusable components that adapt to their context, not just the viewport.” - Heydon Pickering, Web Accessibility Specialist and Author, Smashing Magazine
This evolution fits naturally into component-based design workflows, as seen in examples like responsive card components. While media queries remain essential for managing overall layouts, container queries offer precise control over how individual components behave, making codebases easier to maintain and more reliable.
For Tailwind CSS users, the best approach blends container queries for fine-tuned component behavior with media queries for broader layout adjustments. Although container queries may take some time to master, they pay off by improving modularity and reducing maintenance challenges.
FAQs
How to use container queries in Tailwind?
To use container queries in Tailwind CSS:
- First, add the required plugin to your
tailwind.config.js
file:
plugins: [require('@tailwindcss/container-queries')]
- Then, apply the
@container
class to the parent element. For child elements, use container query classes like@md:text-lg
.
For a detailed example, refer to the Responsive Product Card section mentioned earlier.
How do I enable container queries?
Here’s how you can enable container queries:
- Browser Compatibility: Make sure you’re working with modern browsers that support container queries.
- Steps to Implement:
- Use the
@container
class to define a containment context. - Replace traditional media query classes with the corresponding container query classes.
- Test thoroughly to ensure the design adapts well to different container sizes.
- Use the
For practical guidance, check out the Responsive Product Card example in the earlier sections. Tools like Hoverify can help visualize breakpoints in real time for debugging container queries.